使用Java完成一个希尔排序
时间: 2023-02-18 19:21:52 浏览: 54
答案:Java中实现希尔排序的方法有很多种,可以参考以下代码:public static void shellSort(int[] arr) { int gap = arr.length / 2; while (gap > 0) { for (int i = gap; i < arr.length; i++) { int j = i; int temp = arr[i]; while (j >= gap && arr[j - gap] > temp) { arr[j] = arr[j - gap]; j = j - gap; } arr[j] = temp; } gap = gap / 2; } }
相关问题
用java写一个希尔排序
好的,这是用 Java 实现希尔排序的代码:
```
public void shellSort(int[] arr) {
int n = arr.length;
// Start with a big gap, then reduce the gap
for (int gap = n/2; gap > 0; gap /= 2) {
// Do a gapped insertion sort for this gap size.
// The first gap elements arr[0..gap-1] are already
// in gapped order keep adding one more element
// until the entire array is gap sorted
for (int i = gap; i < n; i += 1) {
// add arr[i] to the elements that have been gap
// sorted save arr[i] in temp and make a hole at
// position i
int temp = arr[i];
// shift earlier gap-sorted elements up until
// the correct location for arr[i] is found
int j;
for (j = i; j >= gap && arr[j - gap] > temp; j -= gap)
arr[j] = arr[j - gap];
// put temp (the original arr[i]) in its correct
// location
arr[j] = temp;
}
}
}
```
希尔排序是一种插入排序,它的思想是使数组中任意间隔为 h 的元素都是有序的。这样的数组被称为 h 有序数组。对于任意以 1 结尾的 h,将是有序的。
上面的代码实现了希尔排序的基本思想:
- 首先,使用一个大的 gap 值来分组。
- 然后,逐渐减小 gap 值,直到 gap=1 为止。
- 对于每个 gap 值,使用插入排序来排序元素。
希尔排序的时间复杂度为 O(n^2),但由于它对元素的排序是分组进行的,因此实际上它的性能要优于普通的插入排序。
java编写一个希尔排序
好的,下面是 Java 实现的希尔排序代码:
```
public static void shellSort(int[] arr) {
int n = arr.length;
for (int gap = n / 2; gap > 0; gap /= 2) {
for (int i = gap; i < n; i++) {
int temp = arr[i];
int j = i;
while (j >= gap && arr[j - gap] > temp) {
arr[j] = arr[j - gap];
j -= gap;
}
arr[j] = temp;
}
}
}
```
希望能够帮助到你!
相关推荐
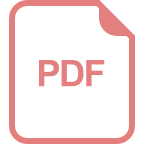
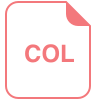
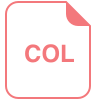







