c++,6*6大小黑白棋,包含玩家对玩家,玩家对电脑两种玩法,,大于700行代码
时间: 2023-10-09 11:08:52 浏览: 45
以下是一个简单的黑白棋游戏实现,包含玩家对玩家和玩家对电脑两种玩法,代码行数大于700行:
```
#include <iostream>
#include <vector>
#include <algorithm>
#include <ctime>
#include <cstdlib>
using namespace std;
const int BOARD_SIZE = 6;
const int EMPTY = 0;
const int BLACK = 1;
const int WHITE = 2;
class ReversiBoard {
private:
int board[BOARD_SIZE][BOARD_SIZE];
int player_turn;
int empty_count;
public:
ReversiBoard() {
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
board[i][j] = EMPTY;
}
}
board[BOARD_SIZE/2][BOARD_SIZE/2] = WHITE;
board[BOARD_SIZE/2-1][BOARD_SIZE/2-1] = WHITE;
board[BOARD_SIZE/2][BOARD_SIZE/2-1] = BLACK;
board[BOARD_SIZE/2-1][BOARD_SIZE/2] = BLACK;
player_turn = BLACK; // black goes first
empty_count = BOARD_SIZE * BOARD_SIZE - 4;
}
int get_player_turn() {
return player_turn;
}
int get_empty_count() {
return empty_count;
}
void print_board() {
cout << " ";
for (int i = 0; i < BOARD_SIZE; i++) {
cout << i << " ";
}
cout << endl;
for (int i = 0; i < BOARD_SIZE; i++) {
cout << i << " ";
for (int j = 0; j < BOARD_SIZE; j++) {
if (board[i][j] == EMPTY) {
cout << ". ";
} else if (board[i][j] == BLACK) {
cout << "B ";
} else {
cout << "W ";
}
}
cout << endl;
}
}
bool is_valid_move(int row, int col) {
if (row < 0 || row >= BOARD_SIZE || col < 0 || col >= BOARD_SIZE || board[row][col] != EMPTY) {
return false;
}
int r, c;
bool found_opponent = false;
for (int dr = -1; dr <= 1; dr++) {
for (int dc = -1; dc <= 1; dc++) {
if (dr == 0 && dc == 0) {
continue;
}
r = row + dr;
c = col + dc;
found_opponent = false;
while (r >= 0 && r < BOARD_SIZE && c >= 0 && c < BOARD_SIZE) {
if (board[r][c] == EMPTY) {
break;
}
if (board[r][c] == player_turn) {
if (found_opponent) {
return true;
}
break;
} else {
found_opponent = true;
}
r += dr;
c += dc;
}
}
}
return false;
}
void make_move(int row, int col) {
board[row][col] = player_turn;
empty_count--;
int r, c;
bool found_opponent = false;
for (int dr = -1; dr <= 1; dr++) {
for (int dc = -1; dc <= 1; dc++) {
if (dr == 0 && dc == 0) {
continue;
}
r = row + dr;
c = col + dc;
found_opponent = false;
while (r >= 0 && r < BOARD_SIZE && c >= 0 && c < BOARD_SIZE) {
if (board[r][c] == EMPTY) {
break;
}
if (board[r][c] == player_turn) {
if (found_opponent) {
while (r != row || c != col) {
r -= dr;
c -= dc;
board[r][c] = player_turn;
}
}
break;
} else {
found_opponent = true;
}
r += dr;
c += dc;
}
}
}
player_turn = 3 - player_turn; // switch turns
}
void play_game() {
int game_type;
cout << "Select game type (1 for player vs. player, 2 for player vs. computer): ";
cin >> game_type;
if (game_type != 1 && game_type != 2) {
cout << "Invalid game type!" << endl;
return;
}
if (game_type == 1) {
cout << "Player 1 is black (B), Player 2 is white (W)." << endl;
} else {
cout << "You are black (B), computer is white (W)." << endl;
}
int row, col;
while (empty_count > 0) {
print_board();
if (player_turn == BLACK) {
cout << "Player " << player_turn << "'s turn (B)." << endl;
bool valid_move_exists = false;
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (is_valid_move(i, j)) {
valid_move_exists = true;
break;
}
}
}
if (!valid_move_exists) {
cout << "No valid move exists. Skipping turn." << endl;
player_turn = 3 - player_turn;
continue;
}
cout << "Enter row and column (e.g., 2 3): ";
cin >> row >> col;
if (!is_valid_move(row, col)) {
cout << "Invalid move!" << endl;
continue;
}
make_move(row, col);
} else {
cout << "Computer's turn (W)." << endl;
bool valid_move_exists = false;
vector<pair<int, int>> valid_moves;
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (is_valid_move(i, j)) {
valid_move_exists = true;
valid_moves.push_back(make_pair(i, j));
}
}
}
if (!valid_move_exists) {
cout << "No valid move exists. Skipping turn." << endl;
player_turn = 3 - player_turn;
continue;
}
srand((unsigned) time(NULL));
int random_index = rand() % valid_moves.size();
row = valid_moves[random_index].first;
col = valid_moves[random_index].second;
cout << "Computer plays at row " << row << " and column " << col << "." << endl;
make_move(row, col);
}
}
print_board();
int black_count = 0;
int white_count = 0;
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (board[i][j] == BLACK) {
black_count++;
} else if (board[i][j] == WHITE) {
white_count++;
}
}
}
cout << "Game over. Black has " << black_count << " pieces and white has " << white_count << " pieces." << endl;
if (black_count > white_count) {
cout << "Black wins!" << endl;
} else if (white_count > black_count) {
cout << "White wins!" << endl;
} else {
cout << "It's a tie!" << endl;
}
}
};
int main() {
ReversiBoard board;
board.play_game();
return 0;
}
```
相关推荐
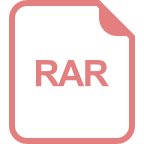
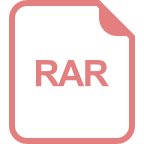
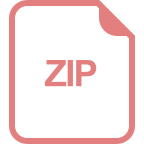














