调用一个函数对一个5×3的矩阵进行转置,并将结果存放在另一个二维数组中,在主函数中输出结果
时间: 2024-11-06 16:18:37 浏览: 24
在C#中,你可以创建一个方法来完成这个操作。首先,你需要定义两个二维数组,一个是原始矩阵,另一个用于存储转置后的矩阵。然后使用`Array.Copy`或者嵌套循环来实现矩阵的转置。
以下是一个简单的例子:
```csharp
using System;
public class MatrixTranspose
{
public static void Main(string[] args)
{
// 定义一个5x3的矩阵
int[,] originalMatrix = new int[5, 3]
{
{1, 2, 3},
{4, 5, 6},
{7, 8, 9},
{10, 11, 12},
{13, 14, 15}
};
// 另一个用来存放转置后的矩阵
int[,]' transposedMatrix = new int[3, 5];
// 转置矩阵
Transpose(originalMatrix, ref transposedMatrix);
// 输出转置后的矩阵
PrintMatrix(transposedMatrix);
}
public static void Transpose(int[,] matrix, ref int[,] result)
{
if (matrix.GetLength(0) != result.GetLength(1))
{
throw new ArgumentException("Original and destination matrices must have compatible dimensions for transpose.");
}
for (int i = 0; i < matrix.GetLength(0); i++)
{
for (int j = 0; j < matrix.GetLength(1); j++)
{
result[j, i] = matrix[i, j];
}
}
}
public static void PrintMatrix(int[,] matrix)
{
Console.WriteLine("Transposed Matrix:");
for (int i = 0; i < matrix.GetLength(0); i++)
{
for (int j = 0; j < matrix.GetLength(1); j++)
{
Console.Write(matrix[i, j] + " ");
}
Console.WriteLine();
}
Console.WriteLine();
}
}
```
在这个示例中,我们定义了一个名为`Transpose`的方法接受一个原始矩阵和一个引用类型的转置矩阵参数,将原矩阵按列转置到新矩阵中。`PrintMatrix`方法则用于打印矩阵的内容。记得运行`Main`函数才能看到结果。如果你有任何关于矩阵转置的问题,请随时提问。
阅读全文
相关推荐
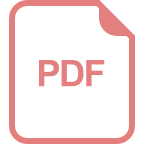
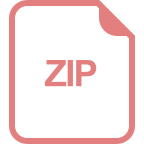















