vue 和vue-konva和konva 使用案例,代码
时间: 2023-08-08 07:08:11 浏览: 67
以下是一个使用 Vue 和 vue-konva 创建画板应用程序的示例代码:
```
<template>
<div>
<v-stage :config="stageSize">
<v-layer>
<v-rect :config="backgroundRect" />
<v-line
v-for="(line, index) in lines"
:key="index"
:config="{
points: line.points,
stroke: 'black',
strokeWidth: 2,
lineJoin: 'round',
lineCap: 'round',
}"
/>
<v-text
v-if="isTyping"
ref="textInput"
:config="{
text: currentText,
x: textPosition.x,
y: textPosition.y - textHeight,
fontSize: textSize,
fill: 'black',
}"
:focus="true"
@input="onTextInput"
@blur="onTextBlur"
/>
</v-layer>
</v-stage>
<button @click="undo">Undo</button>
<button @click="clear">Clear</button>
</div>
</template>
<script>
import VueKonva from 'vue-konva';
export default {
components: {
'v-stage': VueKonva.Stage,
'v-layer': VueKonva.Layer,
'v-rect': VueKonva.Rect,
'v-line': VueKonva.Line,
'v-text': VueKonva.Text,
},
data() {
return {
stageSize: {
width: 500,
height: 500,
},
backgroundRect: {
x: 0,
y: 0,
width: 500,
height: 500,
fill: 'white',
},
lines: [],
isDrawing: false,
isTyping: false,
currentText: '',
textPosition: { x: 0, y: 0 },
textSize: 16,
textHeight: 0,
};
},
methods: {
onMouseDown(event) {
if (event.evt.button === 0) {
this.isDrawing = true;
const pos = this.getRelativePointerPosition();
this.lines.push({
points: [pos.x, pos.y],
});
}
},
onMouseMove(event) {
if (!this.isDrawing) {
return;
}
const pos = this.getRelativePointerPosition();
const lastLine = this.lines[this.lines.length - 1];
lastLine.points.push(pos.x, pos.y);
},
onMouseUp(event) {
this.isDrawing = false;
},
onTextInput(event) {
this.currentText = event.target.value;
this.textHeight = this.$refs.textInput.getTextHeight();
},
onTextBlur(event) {
this.isTyping = false;
const pos = this.getRelativePointerPosition();
this.textPosition = pos;
this.lines.push({
text: this.currentText,
position: pos,
fontSize: this.textSize,
});
this.currentText = '';
},
undo() {
this.lines.pop();
},
clear() {
this.lines = [];
},
getRelativePointerPosition() {
const stage = this.$refs.stage.getStage();
const pointer = stage.getPointerPosition();
const transform = stage.getAbsoluteTransform().copy();
transform.invert();
return transform.point(pointer);
},
},
};
</script>
```
该代码使用了 vue-konva 中的组件来创建矩形、线条、文本等元素,并使用 Vue 的数据绑定功能来实现交互性。
这是一个简单的使用 Konva 创建动态图形的示例代码:
```
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>Konva Example</title>
<script src="https://cdn.jsdelivr.net/npm/konva@8.2.0/konva.min.js"></script>
</head>
<body>
<div id="container"></div>
<script>
const stage = new Konva.Stage({
container: 'container',
width: window.innerWidth,
height: window.innerHeight,
});
const layer = new Konva.Layer();
stage.add(layer);
const circle = new Konva.Circle({
x: stage.width() / 2,
y: stage.height() / 2,
radius: 50,
fill: 'red',
stroke: 'black',
strokeWidth: 4,
});
layer.add(circle);
const anim = new Konva.Animation((frame) => {
const scale = Math.sin(frame.time / 1000) + 1;
circle.scale({ x: scale, y: scale });
}, layer);
anim.start();
</script>
</body>
</html>
```
该代码使用 Konva 中的图形元素和动画功能来创建一个随时间变化的圆形。
相关推荐
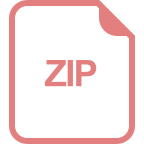
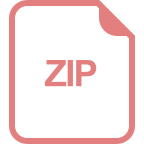
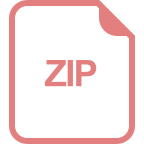














