java根据word模板导出
时间: 2023-07-31 13:13:44 浏览: 66
要根据Word模板导出Java代码,可以使用Apache POI和FreeMarker这两个开源库来实现。具体步骤如下:
1. 使用Apache POI读取Word模板文件,获取模板中的内容。
2. 将读取到的内容传递给FreeMarker进行解析,生成需要填充的数据。
3. 将生成的数据填充到Word模板中,生成最终的Word文档。
以下是实现的简单示例代码:
```
// 读取Word模板文件
FileInputStream fis = new FileInputStream("template.docx");
XWPFDocument doc = new XWPFDocument(fis);
// 获取模板中的内容
List<XWPFParagraph> paragraphs = doc.getParagraphs();
List<XWPFTable> tables = doc.getTables();
// 使用FreeMarker生成数据
Configuration cfg = new Configuration(Configuration.VERSION_2_3_0);
cfg.setDefaultEncoding("UTF-8");
cfg.setClassForTemplateLoading(this.getClass(), "/templates");
Map<String, Object> data = new HashMap<>();
data.put("name", "张三");
data.put("age", 20);
Template template = cfg.getTemplate("template.ftl");
StringWriter writer = new StringWriter();
template.process(data, writer);
// 将生成的数据填充到Word模板中
String content = writer.toString();
for (XWPFParagraph paragraph : paragraphs) {
String text = paragraph.getText();
if (text.contains("${")) {
text = text.replaceAll("\\$\\{.*?\\}", content);
paragraph.setText(text);
}
}
for (XWPFTable table : tables) {
List<XWPFTableRow> rows = table.getRows();
for (XWPFTableRow row : rows) {
List<XWPFTableCell> cells = row.getTableCells();
for (XWPFTableCell cell : cells) {
String text = cell.getText();
if (text.contains("${")) {
text = text.replaceAll("\\$\\{.*?\\}", content);
cell.setText(text);
}
}
}
}
// 保存最终的Word文档
FileOutputStream fos = new FileOutputStream("output.docx");
doc.write(fos);
fos.close();
```
以上代码仅供参考,具体实现还需要根据实际需求进行调整。
相关推荐
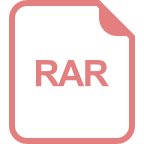
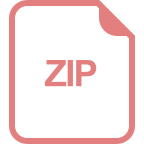
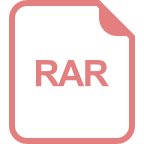
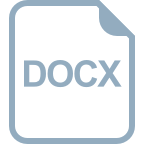
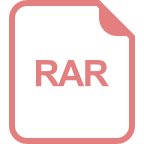
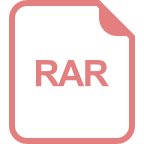
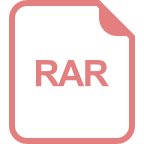
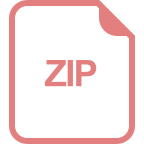
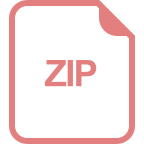
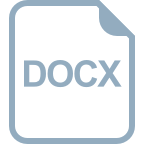
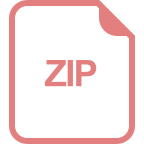
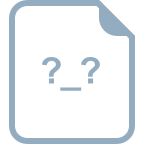
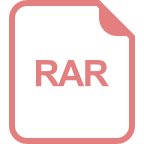