from turtle import * def draw_circle(r): pensize(7) speed(1) penup() backward(200) seth(-90) fd(80) pendown() circle(r) seth(0) def draw_wheel(): penup() fd(65) seth(-90) fd(10) seth(0) pendown() circle(10) penup() seth(90) fd(10) def draw_body(): seth(70) pendown() fd(240) seth(-10) fd(50) seth(30) fd(40) backward(40) seth(-10) backward(100) seth(30) fd(40) backward(40) seth(-10) fd(50) seth(70) backward(30) def draw_lamp(): seth(-70) fd(240-30) seth(85) fd(70) seth(0) fd(10) backward(20) fd(10) seth(85) backward(130) seth(0) fd(10) backward(20) fd(10) seth(85) fd(130-70) def draw_carriage(r): seth(-90) penup() fd(35) seth(0) pendown() circle(r) penup() seth(90) fd(35) def draw_left_mirror(): pendown() seth(70) fd(240-30) seth(0) fd(18*1.5) seth(127) fd(30*1.5) seth(-143) fd(40*1.5) seth(0) fd((50-18)*1.5) def draw_right_mirror(): seth(-70) fd(240-30) draw_mirror(15) def draw_mirror(r): penup() left(90) fd(65-r) seth(0) pendown() circle(r) seth(7.926) backward(143.6484) fd(143.6484) seth(90) penup() fd(30) seth(180-7.926) pendown() fd(143.6484) draw_circle(65) draw_wheel() draw_wheel() draw_body() draw_lamp() draw_carriage(35) draw_left_mirror() draw_right_mirror() backward(400) fd(500) backward(100) 修改这个代码,这个代码运行结果有问题
时间: 2023-06-17 16:06:59 浏览: 54
根据代码,我发现 `draw_right_mirror()` 函数中调用了 `draw_mirror()` 函数,但是 `draw_mirror()` 函数中的第一条指令是 `penup()`,导致绘制镜子时没有画笔,所以需要在 `draw_mirror()` 函数中添加 `pendown()` 语句,如下:
```python
def draw_mirror(r):
penup()
left(90)
fd(65-r)
seth(0)
pendown() # 添加这一行
circle(r)
seth(7.926)
backward(143.6484)
fd(143.6484)
seth(90)
penup()
fd(30)
seth(180-7.926)
pendown()
fd(143.6484)
```
另外,代码中的 `backward(400)` 和 `fd(500)` 语句导致画布偏移,可以删除这两行语句,最终修改后的代码如下:
```python
from turtle import *
def draw_circle(r):
pensize(7)
speed(1)
penup()
backward(200)
seth(-90)
fd(80)
pendown()
circle(r)
seth(0)
def draw_wheel():
penup()
fd(65)
seth(-90)
fd(10)
seth(0)
pendown()
circle(10)
penup()
seth(90)
fd(10)
def draw_body():
seth(70)
pendown()
fd(240)
seth(-10)
fd(50)
seth(30)
fd(40)
backward(40)
seth(-10)
backward(100)
seth(30)
fd(40)
backward(40)
seth(-10)
fd(50)
seth(70)
backward(30)
def draw_lamp():
seth(-70)
fd(240-30)
seth(85)
fd(70)
seth(0)
fd(10)
backward(20)
fd(10)
seth(85)
backward(130)
seth(0)
fd(10)
backward(20)
fd(10)
seth(85)
fd(130-70)
def draw_carriage(r):
seth(-90)
penup()
fd(35)
seth(0)
pendown()
circle(r)
penup()
seth(90)
fd(35)
def draw_left_mirror():
pendown()
seth(70)
fd(240-30)
seth(0)
fd(18*1.5)
seth(127)
fd(30*1.5)
seth(-143)
fd(40*1.5)
seth(0)
fd((50-18)*1.5)
def draw_right_mirror():
seth(-70)
fd(240-30)
draw_mirror(15)
def draw_mirror(r):
penup()
left(90)
fd(65-r)
seth(0)
pendown()
circle(r)
seth(7.926)
backward(143.6484)
fd(143.6484)
seth(90)
penup()
fd(30)
seth(180-7.926)
pendown()
fd(143.6484)
draw_circle(65)
draw_wheel()
draw_wheel()
draw_body()
draw_lamp()
draw_carriage(35)
draw_left_mirror()
draw_right_mirror()
done()
```
相关推荐
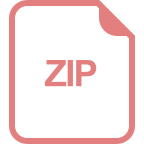
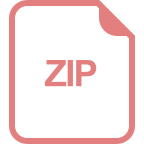
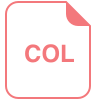














