java 根据前端传表名和查询条件,后端获取指定表中数据
时间: 2023-06-16 19:02:12 浏览: 96
你可以使用 JDBC(Java 数据库连接) API 来连接数据库,并根据前端传递的表名和查询条件获取指定表中的数据。以下是一个简单的示例代码:
```
import java.sql.*;
public class DataAccess {
// 数据库连接信息
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost:3306/mydatabase";
static final String USER = "root";
static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 注册 JDBC 驱动
Class.forName(JDBC_DRIVER);
// 打开连接
conn = DriverManager.getConnection(DB_URL, USER, PASS);
// 执行查询
String tableName = "mytable";
String condition = "age > 18";
String sql = "SELECT * FROM " + tableName + " WHERE " + condition;
stmt = conn.createStatement();
rs = stmt.executeQuery(sql);
// 处理结果集
while (rs.next()) {
// 获取每一行的数据
String name = rs.getString("name");
int age = rs.getInt("age");
System.out.println("Name: " + name + ", Age: " + age);
}
} catch (SQLException se) {
// 处理 JDBC 错误
se.printStackTrace();
} catch (Exception e) {
// 处理 Class.forName 错误
e.printStackTrace();
} finally {
// 关闭资源
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException se) {
se.printStackTrace();
}
}
}
}
```
在上面的示例代码中,我们使用了 JDBC 驱动来连接 MySQL 数据库,并根据前端传递的表名和查询条件执行了查询操作。你可以根据自己的需要进行修改。
相关推荐
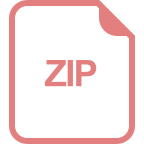
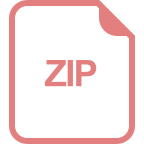
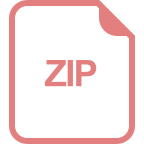














