fabric java sdk spring boot项目详解
时间: 2023-08-01 15:11:52 浏览: 72
Fabric Java SDK 是为 Hyperledger Fabric 区块链平台开发的 Java SDK,它提供了访问 Fabric 网络的所有必要接口,包括身份验证、链码调用、交易提交等。Spring Boot 是一个基于 Spring 框架的快速开发应用程序的工具,它提供了诸多便利的特性,如自动配置、快速开发等。
在使用 Fabric Java SDK 开发 Spring Boot 项目时,我们可以采用以下步骤:
1. 配置 Maven 依赖
在 pom.xml 文件中添加以下依赖:
```xml
<dependency>
<groupId>org.hyperledger.fabric-sdk-java</groupId>
<artifactId>fabric-sdk-java</artifactId>
<version>2.2.0</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
2. 配置 Fabric SDK
在 Spring Boot 项目中,我们可以使用 @Configuration 注解配置 Fabric SDK。在配置中,我们需要指定以下参数:
- Fabric 网络的 CA 证书
- Fabric 网络的 MSP 证书
- Fabric 网络的连接配置文件
```java
@Configuration
public class FabricConfig {
private static final String CONNECTION_PROFILE_PATH = "connection-profile.yaml";
private static final String MSP_PATH = "/msp";
private static final String CA_ORG1_URL = "http://localhost:7054";
private static final String CHANNEL_NAME = "mychannel";
@Bean
public NetworkConfig getNetworkConfig() throws Exception {
Path connectionProfilePath = Paths.get(CONNECTION_PROFILE_PATH);
return NetworkConfig.fromYamlFile(connectionProfilePath.toFile());
}
@Bean
public User getUser(NetworkConfig networkConfig) throws Exception {
UserContext userContext = new UserContext();
userContext.setName("user1");
userContext.setAffiliation("org1");
userContext.setMspId("Org1MSP");
Path mspPath = Paths.get(networkConfig.getOrganizationInfo("Org1").getMspId(), MSP_PATH);
userContext.setEnrollment(new Enrollment() {
@Override
public PrivateKey getKey() {
try {
return loadPrivateKey(mspPath);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
@Override
public String getCert() {
try {
return new String(loadCert(mspPath), "UTF-8");
} catch (Exception e) {
throw new RuntimeException(e);
}
}
});
return userContext;
}
@Bean
public FabricGateway getGateway(NetworkConfig networkConfig, User user) throws Exception {
Gateway.Builder builder = Gateway.createBuilder();
builder.identity(user);
builder.networkConfig(networkConfig);
return builder.connect().get();
}
@Bean
public Contract getContract(FabricGateway gateway) throws Exception {
return gateway.getNetwork(CHANNEL_NAME).getContract("mychaincode");
}
private byte[] loadCert(Path mspPath) throws Exception {
Path certPath = mspPath.resolve(Paths.get("signcerts", "cert.pem"));
return Files.readAllBytes(certPath);
}
private PrivateKey loadPrivateKey(Path mspPath) throws Exception {
Path keyPath = mspPath.resolve(Paths.get("keystore", "priv.key"));
byte[] keyBytes = Files.readAllBytes(keyPath);
return getPrivateKeyFromBytes(keyBytes);
}
private PrivateKey getPrivateKeyFromBytes(byte[] keyBytes) throws Exception {
PKCS8EncodedKeySpec spec = new PKCS8EncodedKeySpec(keyBytes);
KeyFactory factory = KeyFactory.getInstance("EC");
return factory.generatePrivate(spec);
}
}
```
3. 实现接口方法
在接口方法中,我们可以使用 Fabric SDK 提供的 API 实现对链码的调用、交易的提交等操作。
```java
@RestController
public class MyController {
private static final String CHAINCODE_NAME = "mychaincode";
private static final String FUNCTION_NAME = "invoke";
private static final String KEY = "key1";
private static final String VALUE = "value1";
@Autowired
private Contract contract;
@PostMapping("/set")
public String setValue() throws Exception {
contract.submitTransaction(FUNCTION_NAME, KEY, VALUE);
return "success";
}
@GetMapping("/get")
public String getValue() throws Exception {
byte[] result = contract.evaluateTransaction(FUNCTION_NAME, KEY);
return new String(result, "UTF-8");
}
}
```
以上就是使用 Fabric Java SDK 开发 Spring Boot 项目的流程。通过这种方式,我们可以方便地实现对 Fabric 网络的访问,并快速开发出符合需求的应用程序。
相关推荐
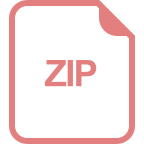
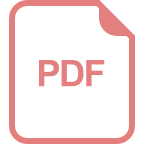
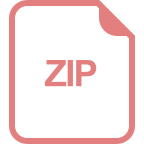














