Java:分别利用 synchronized、Lock和阻塞队列的方法模拟生产者-消费者的同步问题。 分别利用 synchronized、Lock和阻塞队列的方法实现交替输出ABABABAB。
时间: 2024-03-14 07:44:15 浏览: 18
生产者-消费者同步问题的实现:
1. 使用 synchronized:
```java
public class ProducerConsumerWithSync {
private static final int CAPACITY = 5;
private static Queue<Integer> queue = new LinkedList<>();
public static void main(String[] args) {
Thread producer = new Producer();
Thread consumer = new Consumer();
producer.start();
consumer.start();
}
static class Producer extends Thread {
@Override
public void run() {
synchronized (queue) {
while (true) {
while (queue.size() == CAPACITY) {
try {
queue.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
int num = new Random().nextInt();
queue.offer(num);
System.out.println("Produced " + num);
queue.notifyAll();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
static class Consumer extends Thread {
@Override
public void run() {
synchronized (queue) {
while (true) {
while (queue.isEmpty()) {
try {
queue.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
int num = queue.poll();
System.out.println("Consumed " + num);
queue.notifyAll();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
}
```
2. 使用 Lock:
```java
public class ProducerConsumerWithLock {
private static final int CAPACITY = 5;
private static Queue<Integer> queue = new LinkedList<>();
private static Lock lock = new ReentrantLock();
private static Condition notFull = lock.newCondition();
private static Condition notEmpty = lock.newCondition();
public static void main(String[] args) {
Thread producer = new Producer();
Thread consumer = new Consumer();
producer.start();
consumer.start();
}
static class Producer extends Thread {
@Override
public void run() {
while (true) {
lock.lock();
try {
while (queue.size() == CAPACITY) {
notFull.await();
}
int num = new Random().nextInt();
queue.offer(num);
System.out.println("Produced " + num);
notEmpty.signalAll();
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
}
}
static class Consumer extends Thread {
@Override
public void run() {
while (true) {
lock.lock();
try {
while (queue.isEmpty()) {
notEmpty.await();
}
int num = queue.poll();
System.out.println("Consumed " + num);
notFull.signalAll();
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
}
}
}
```
3. 使用阻塞队列:
```java
public class ProducerConsumerWithBlockingQueue {
private static final int CAPACITY = 5;
private static BlockingQueue<Integer> queue = new ArrayBlockingQueue<>(CAPACITY);
public static void main(String[] args) {
Thread producer = new Producer();
Thread consumer = new Consumer();
producer.start();
consumer.start();
}
static class Producer extends Thread {
@Override
public void run() {
while (true) {
try {
int num = new Random().nextInt();
queue.put(num);
System.out.println("Produced " + num);
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
static class Consumer extends Thread {
@Override
public void run() {
while (true) {
try {
int num = queue.take();
System.out.println("Consumed " + num);
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
```
交替输出 ABABABAB 的实现:
1. 使用 synchronized:
```java
public class AlternatePrintWithSync {
private static final Object lock = new Object();
private static boolean flag = true;
public static void main(String[] args) {
Thread thread1 = new Thread(new PrintThread(1), "Thread 1");
Thread thread2 = new Thread(new PrintThread(2), "Thread 2");
thread1.start();
thread2.start();
}
static class PrintThread implements Runnable {
private int num;
public PrintThread(int num) {
this.num = num;
}
@Override
public void run() {
for (int i = 0; i < 4; i++) {
synchronized (lock) {
while ((num == 1 && !flag) || (num == 2 && flag)) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println(Thread.currentThread().getName() + ": " + (char) ('A' + i));
flag = !flag;
lock.notifyAll();
}
}
}
}
}
```
2. 使用 Lock:
```java
public class AlternatePrintWithLock {
private static final Lock lock = new ReentrantLock();
private static final Condition condition1 = lock.newCondition();
private static final Condition condition2 = lock.newCondition();
private static boolean flag = true;
public static void main(String[] args) {
Thread thread1 = new Thread(new PrintThread(1), "Thread 1");
Thread thread2 = new Thread(new PrintThread(2), "Thread 2");
thread1.start();
thread2.start();
}
static class PrintThread implements Runnable {
private int num;
public PrintThread(int num) {
this.num = num;
}
@Override
public void run() {
for (int i = 0; i < 4; i++) {
lock.lock();
try {
if ((num == 1 && !flag) || (num == 2 && flag)) {
if (num == 1) {
condition1.await();
} else {
condition2.await();
}
}
System.out.println(Thread.currentThread().getName() + ": " + (char) ('A' + i));
flag = !flag;
if (num == 1) {
condition2.signalAll();
} else {
condition1.signalAll();
}
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
}
}
}
```
3. 使用阻塞队列:
```java
public class AlternatePrintWithBlockingQueue {
private static BlockingQueue<Integer> queue1 = new ArrayBlockingQueue<>(1);
private static BlockingQueue<Integer> queue2 = new ArrayBlockingQueue<>(1);
public static void main(String[] args) {
Thread thread1 = new Thread(new PrintThread(1), "Thread 1");
Thread thread2 = new Thread(new PrintThread(2), "Thread 2");
thread1.start();
thread2.start();
}
static class PrintThread implements Runnable {
private int num;
public PrintThread(int num) {
this.num = num;
}
@Override
public void run() {
for (int i = 0; i < 4; i++) {
try {
if (num == 1) {
queue1.put(1);
} else {
queue2.put(1);
}
System.out.println(Thread.currentThread().getName() + ": " + (char) ('A' + i));
if (num == 1) {
queue2.take();
} else {
queue1.take();
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
```
相关推荐
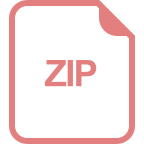
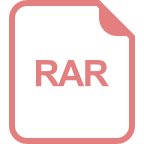
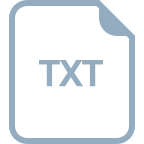
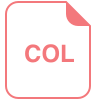
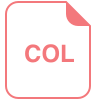
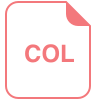
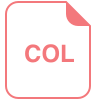
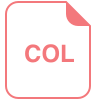









