uniapp实现在一个输入框按回车键后光标跳到下一个输入框
时间: 2024-04-28 08:23:03 浏览: 9
您可以在 `input` 标签中监听 `keydown` 事件,当用户按下回车键时,通过 `nextTick` 方法将光标聚焦到下一个输入框中。
以下是一个简单的示例代码:
```html
<template>
<div>
<input ref="input1" type="text" @keydown.enter="nextInput">
<input ref="input2" type="text">
</div>
</template>
<script>
export default {
methods: {
nextInput() {
this.$nextTick(() => {
this.$refs.input2.focus()
})
}
}
}
</script>
```
在上面的示例中,当用户在第一个输入框中按下回车键时,`nextInput` 方法会被调用,该方法通过 `$nextTick` 方法将光标聚焦到第二个输入框中。
请注意,上述示例仅适用于两个输入框的情况。如果您需要处理多个输入框的情况,可以考虑将 `nextInput` 方法改为可配置的,以便于在不同的输入框中使用。
相关问题
java输入值按回车下一个_java 如何 按回车 光标换到下个输入框
可以使用Java Swing中的JTextField和ActionListener实现这个功能。具体实现步骤如下:
1. 创建一个JFrame窗口和多个JTextField输入框,用于输入不同的值。
2. 为每个JTextField添加ActionListener,当用户按下回车键时,将焦点转移到下一个JTextField输入框。
3. 在ActionListener中使用requestFocus()方法将焦点转移到下一个JTextField。
下面是一个简单的示例代码:
```
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JFrame;
import javax.swing.JTextField;
public class InputDemo extends JFrame implements ActionListener {
private JTextField input1, input2, input3;
public InputDemo() {
super("Input Demo");
setLayout(new FlowLayout());
input1 = new JTextField(10);
input2 = new JTextField(10);
input3 = new JTextField(10);
input1.addActionListener(this);
input2.addActionListener(this);
input3.addActionListener(this);
add(input1);
add(input2);
add(input3);
setSize(300, 100);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == input1) {
input2.requestFocus();
} else if (e.getSource() == input2) {
input3.requestFocus();
} else if (e.getSource() == input3) {
// do something with the inputs
}
}
public static void main(String[] args) {
InputDemo demo = new InputDemo();
}
}
```
在这个示例中,当用户在第一个输入框中按下回车键时,焦点会转移到第二个输入框;当用户在第二个输入框中按下回车键时,焦点会转移到第三个输入框。你可以根据需要修改代码以满足你的需求。
vue3实现一个输入框光标位置插入指定字符
可以使用 `ref` 获取输入框的 DOM 元素,然后在插入字符的时候获取光标位置并插入字符。具体实现可以参考以下代码:
```
<template>
<div>
<input ref="input" type="text" v-model="text" @keydown.enter.prevent="insertChar">
</div>
</template>
<script>
import { ref } from 'vue';
export default {
setup() {
const text = ref('');
// 获取输入框的 DOM 元素
const inputRef = ref(null);
const inputEl = inputRef.value;
// 插入指定字符
const insertChar = () => {
const startPos = inputEl.selectionStart;
const endPos = inputEl.selectionEnd;
const char = 'X';
text.value = text.value.substring(0, startPos) + char + text.value.substring(endPos, text.value.length);
inputEl.selectionStart = inputEl.selectionEnd = startPos + 1;
};
return {
text,
inputRef,
insertChar
};
}
};
</script>
```
在上面的代码中,我们使用 `ref` 创建了一个名为 `inputRef` 的引用,然后通过 `inputRef.value` 获取到输入框的 DOM 元素。在 `insertChar` 方法中,我们先获取当前的光标位置 `startPos` 和 `endPos`,然后插入指定字符,最后将光标位置设置到插入的字符后面。
需要注意的是,在模板中我们使用了 `@keydown.enter.prevent` 修饰符来阻止回车键默认事件,以便在按下回车键时执行 `insertChar` 方法。
相关推荐










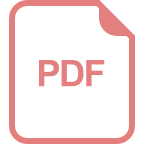