如何用纯C语言在Linux环境下开发一个串口通信程序,用于数据的接收和发送?附带完整代码
时间: 2024-10-20 15:05:05 浏览: 26
在Linux环境下使用C语言开发串口通信程序,你需要使用`stdio.h`、`fcntl.h`、`termios.h` 和 `unistd.h` 等头文件,它们提供了对终端控制台和串口操作的访问。以下是一个简单的步骤和示例代码:
1. 打开串口设备:
```c
#include <stdio.h>
#include <fcntl.h>
int open_serial_port(const char *port_name, int baud_rate) {
int fd = open(port_name, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("Error opening serial port");
return -1;
}
struct termios options;
bzero(&options, sizeof(options));
cfsetispeed(&options, baud_rate);
cfsetospeed(&options, baud_rate);
tcgetattr(fd, &options);
cfmakeraw(&options); // 开启无校验模式
// 设置其他选项,如波特率、数据位、停止位等
options.c_cflag &= ~CSIZE; // 清除数据位标志
options.c_cflag |= CS8; // 设置为8位数据
options.c_iflag &= ~(IXON | IXOFF | IXANY); // 关闭流量控制
tcflush(fd, TCIFLUSH); // 清空输入缓冲区
tcsetattr(fd, TCSANOW, &options);
return fd;
}
```
2. 数据收发:
```c
void send_data(int fd, const char *data, size_t len) {
write(fd, data, len);
}
void receive_data(int fd, char *buffer, size_t buf_len) {
ssize_t bytes_received = read(fd, buffer, buf_len);
if (bytes_received > 0) {
printf("Received %zd bytes: %s\n", bytes_received, buffer);
} else {
perror("Error receiving data");
}
}
```
3. 主函数示例:
```c
int main() {
int fd = open_serial_port("/dev/ttyS0", B9600); // 指定端口号和波特率
if (fd == -1) {
return 1;
}
char tx_buffer[] = "Hello from C!";
char rx_buffer[100];
send_data(fd, tx_buffer, strlen(tx_buffer));
receive_data(fd, rx_buffer, sizeof(rx_buffer));
close(fd);
return 0;
}
```
记得替换`"/dev/ttyS0"`为实际的串口路径。
**
阅读全文
相关推荐
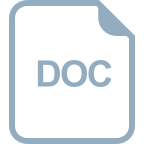
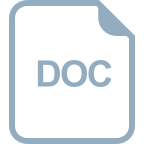
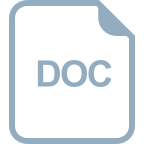
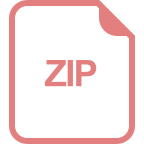
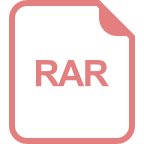
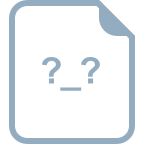
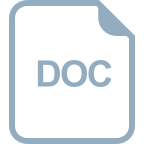
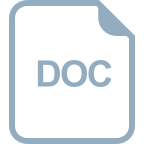
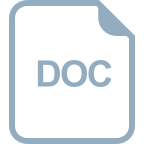
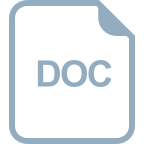
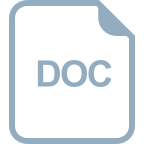
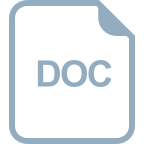
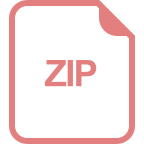
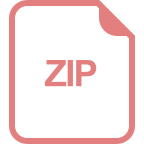
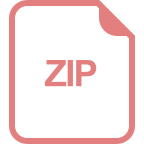
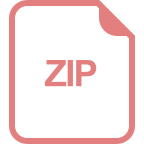
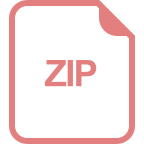
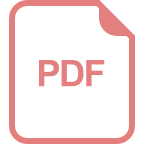
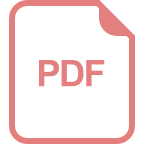