请用python完成这个项目
时间: 2024-12-06 09:27:31 浏览: 9
要使用Python完成这个数字信号处理(DSP)项目的任务,你需要按照以下步骤进行:
### 1. 创建FIR滤波器系数计算函数
首先,创建一个函数来计算和返回FIR滤波器的系数。该函数将使用sinc函数来生成高通和带阻滤波器的组合。
```python
import numpy as np
def calculate_fir_coefficients(fs, fc_highpass, fc_bandstop):
"""
计算FIR滤波器系数
:param fs: 采样率 (Hz)
:param fc_highpass: 高通滤波器截止频率 (Hz)
:param fc_bandstop: 带阻滤波器截止频率 (Hz)
:return: FIR滤波器系数
"""
# 高通滤波器部分
N = 51 # 滤波器阶数
n = np.arange(N)
h_highpass = (1 - np.cos(2 * np.pi * fc_highpass / fs)) * np.sinc(2 * fc_highpass * (n - (N - 1) / 2) / fs)
# 带阻滤波器部分
h_bandstop = -np.sinc(2 * fc_bandstop[0] * (n - (N - 1) / 2) / fs) + np.sinc(2 * fc_bandstop[1] * (n - (N - 1) / 2) / fs)
# 组合滤波器
h_combined = h_highpass + h_bandstop
# 归一化
h_combined /= np.sum(h_combined)
return h_combined
```
### 2. 实现FIR滤波器类
接下来,实现一个FIR滤波器类,该类可以逐样本处理数据以实现实时处理。
```python
class FIRFilter:
def __init__(self, coefficients):
self.coefficients = coefficients
self.delay_line = np.zeros(len(coefficients))
def dofilter(self, value):
self.delay_line = np.roll(self.delay_line, 1)
self.delay_line[0] = value
output = np.dot(self.delay_line, self.coefficients)
return output
```
### 3. 使用LMS自适应滤波器
实现一个LMS自适应滤波器方法,用于去除DC偏移和50Hz噪声。
```python
class AdaptiveFIRFilter(FIRFilter):
def __init__(self, coefficients):
super().__init__(coefficients)
def doFilterAdaptive(self, signal, noise, learning_rate):
error = signal - self.dofilter(noise)
self.coefficients += learning_rate * error * noise
return error
```
### 4. 心跳检测
最后,实现心跳检测功能,检测R峰并计算瞬时心率。
```python
import matplotlib.pyplot as plt
def detect_r_peaks(filtered_ecg, threshold=0.5):
r_peaks = []
for i in range(1, len(filtered_ecg) - 1):
if filtered_ecg[i] > threshold and filtered_ecg[i] > filtered_ecg[i - 1] and filtered_ecg[i] > filtered_ecg[i + 1]:
r_peaks.append(i)
return r_peaks
def plot_heart_rate(r_peaks, fs):
heart_rates = [fs / (r_peaks[i+1] - r_peaks[i]) for i in range(len(r_peaks) - 1)]
times = [r_peaks[i] / fs for i in range(len(r_peaks) - 1)]
plt.plot(times, heart_rates)
plt.xlabel('Time (s)')
plt.ylabel('Heart Rate (BPM)')
plt.title('Instantaneous Heart Rate')
plt.show()
```
### 主程序
将上述所有部分整合到主程序中,并加载ECG数据进行处理。
```python
import scipy.io as sio
# 加载ECG数据
ecg_data = sio.loadmat('ecg.mat') # 根据实际情况修改文件路径
ecg_signal = ecg_data['ecg_signal'].flatten()
fs = 250 # 采样率 (Hz)
# 计算FIR滤波器系数
fc_highpass = 0.5 # 高通滤波器截止频率 (Hz)
fc_bandstop = [49, 51] # 带阻滤波器截止频率 (Hz)
coefficients = calculate_fir_coefficients(fs, fc_highpass, fc_bandstop)
# 创建FIR滤波器实例
fir_filter = FIRFilter(coefficients)
# 过滤ECG信号
filtered_ecg = [fir_filter.dofilter(sample) for sample in ecg_signal]
# 使用LMS自适应滤波器去除DC和50Hz噪声
adaptive_fir_filter = AdaptiveFIRFilter(coefficients)
reference_signal = np.sin(2 * np.pi * 50 * np.arange(len(ecg_signal)) / fs)
cleaned_ecg = [adaptive_fir_filter.doFilterAdaptive(signal, ref, learning_rate=0.01) for signal, ref in zip(filtered_ecg, reference_signal)]
# 检测R峰
r_peaks = detect_r_peaks(cleaned_ecg)
# 绘制心率图
plot_heart_rate(r_peaks, fs)
# 绘制原始和过滤后的ECG信号
plt.figure(figsize=(12, 6))
plt.subplot(2, 1, 1)
plt.plot(ecg_signal)
plt.title('Original ECG Signal')
plt.subplot(2, 1, 2)
plt.plot(cleaned_ecg)
plt.title('Filtered ECG Signal')
plt.tight_layout()
plt.show()
```
### 提交要求
确保提交的报告包括以下内容:
- 完整的Python代码
- ECG信号在时域和频域的图表
- 对每个步骤的详细解释
- 图表应使用矢量图形格式(如EPS、SVG、PDF或EMF)
希望这些代码和说明能帮助你顺利完成项目!如果有任何问题,请随时提问。
阅读全文
相关推荐
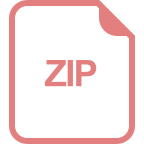
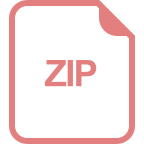
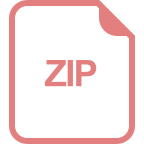
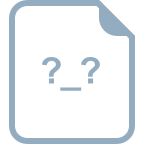
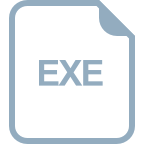
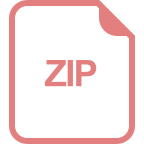
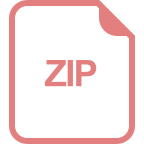
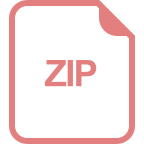
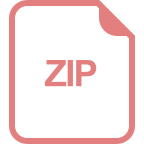
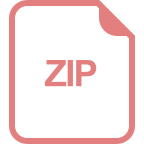
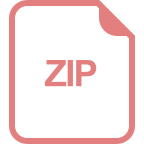
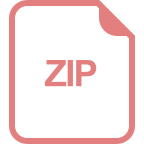
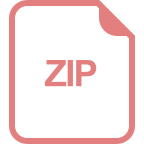
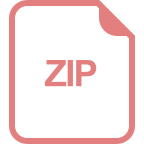
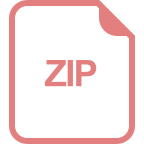
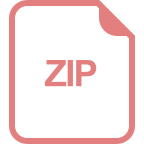