JAVA 有个Map<String,List<String>> 写个方法 获取所有List<String> 拼成一个List
时间: 2023-07-09 17:37:49 浏览: 48
可以使用Java 8的Stream API来实现这个方法,具体代码如下:
```java
public static List<String> getAllLists(Map<String, List<String>> map) {
return map.values()
.stream()
.flatMap(Collection::stream)
.collect(Collectors.toList());
}
```
这个方法接收一个 `Map<String, List<String>>` 类型的参数,返回一个类型为 `List<String>` 的结果。在方法体内,我们首先使用 `values()` 方法获取到参数 `map` 中所有的 `List<String>`,然后使用 `flatMap()` 方法将多个 `List` 合并成一个流,最后使用 `collect()` 方法将这个流转换成一个 `List<String>` 类型的结果。
相关问题
写一个Java方法把List<Map<String, Object>> 转 JSONArray
这里是一个用Java编写的方法,用于将List<Map<String, Object>>转换为JSONArray:
```java
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
public JSONArray convertListMapToJsonArray(List<Map<String, Object>> list) {
JSONArray jsonArray = new JSONArray();
for (Map<String, Object> map : list) {
JSONObject jsonObj = new JSONObject();
for (Map.Entry<String, Object> entry : map.entrySet()) {
jsonObj.put(entry.getKey(), entry.getValue());
}
jsonArray.add(jsonObj);
}
return jsonArray;
}
```
说明:
- `convertListMapToJsonArray`方法接收一个List<Map<String, Object>>参数`list`,表示要转换为JSONArray的数据;
- 方法内部遍历List中的每个Map对象,将Map对象转换为JSONObject对象,并将其添加到JSONArray中;
- 最终返回转换后的JSONArray。
使用该方法时,需要先引入FastJSON库:
```xml
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.75</version>
</dependency>
```
java List<Map<String,String>> 转成 List<Map>
你可以使用Java的原生类型推断来实现List<Map<String, String>>转为List<Map>。在Java 7之后,你可以使用菱形语法来简化泛型类型的定义。下面是一个示例代码:
```java
List<Map<String, String>> originalList = new ArrayList<>();
// 假设originalList已经包含了一些数据
// 使用原生类型推断,将List<Map<String, String>>转为List<Map>
List<Map> newList = new ArrayList<>(originalList);
```
请注意,这种转换会导致Map中的值丢失其具体的数据类型,因为Map的值类型被擦除为Object。如果你需要保留具体的数据类型,可以考虑使用更具体的泛型类型,如List<Map<String, Object>>来代替List<Map>。
相关推荐
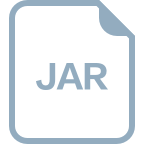
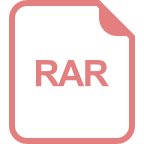












