用<script setup lang="ts">写个tabs组件,并且写出使用方法
时间: 2024-02-14 07:10:17 浏览: 33
好的,以下是使用`<script setup lang="ts">`语法来实现一个tabs组件的示例,并提供使用方法:
```vue
<template>
<div class="tabs">
<div class="tabs-nav">
<div
v-for="(tab, index) in tabs"
:key="index"
:class="{ active: activeTab === index }"
@click="activeTab = index"
>
{{ tab.label }}
</div>
</div>
<div class="tabs-content">
<slot :name="tabs[activeTab].name"></slot>
</div>
</div>
</template>
<script setup lang="ts">
import { defineProps, ref } from 'vue'
interface Tab {
label: string,
name: string,
}
const props = defineProps({
tabs: {
type: Array as () => Tab[],
required: true,
},
})
const activeTab = ref(0)
</script>
<style scoped>
.tabs {
display: flex;
flex-direction: column;
height: 100%;
}
.tabs-nav {
display: flex;
height: 40px;
border-bottom: 1px solid #ccc;
}
.tabs-nav > div {
flex: 1;
display: flex;
justify-content: center;
align-items: center;
cursor: pointer;
}
.tabs-nav > div.active {
color: #333;
font-weight: bold;
border-bottom: 2px solid #333;
}
.tabs-content {
flex: 1;
overflow: auto;
}
</style>
```
使用方法:
```vue
<template>
<tabs :tabs="tabs">
<template #tab1>
<div>Tab 1 Content</div>
</template>
<template #tab2>
<div>Tab 2 Content</div>
</template>
<template #tab3>
<div>Tab 3 Content</div>
</template>
</tabs>
</template>
<script setup lang="ts">
import Tabs from './Tabs.vue'
const tabs = [
{ label: 'Tab 1', name: 'tab1' },
{ label: 'Tab 2', name: 'tab2' },
{ label: 'Tab 3', name: 'tab3' },
]
</script>
```
在上面的示例中,我们使用了`<template>`标签来为每个tab定义内容,并使用`<slot>`标签来动态地显示当前激活的tab的内容。在`<script setup>`语法中,我们使用`defineProps`函数来定义`tabs`这个prop,并且使用`ref`函数来定义一个`activeTab`变量,用来保存当前激活的tab的索引。最后,我们在模板中使用了`v-for`指令来遍历每个tab,并根据`activeTab`的值来判断当前tab是否处于激活状态。
希望这个示例能帮助您实现一个简单的tabs组件,并且提供了对`<script setup>`语法的使用方法。
相关推荐
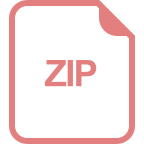
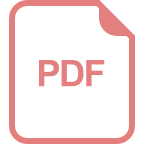
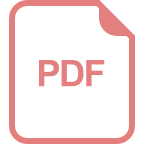








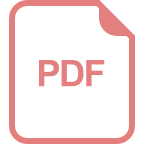
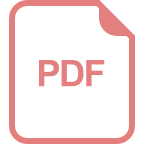
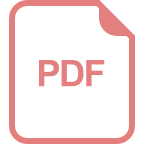
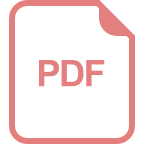
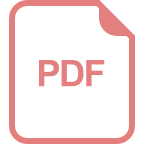
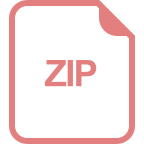