操作系统吸烟者问题C语言代码
时间: 2023-12-20 11:01:15 浏览: 33
下面是一个基于信号量的解法的C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#include <unistd.h>
#define N 3 // 最大吸烟者数量
sem_t tobacco; // 烟草
sem_t paper; // 纸
sem_t matches; // 火柴
sem_t agent; // 客服
void* smoker_tobacco(void* arg); // 吸烟者1
void* smoker_paper(void* arg); // 吸烟者2
void* smoker_matches(void* arg); // 吸烟者3
void* agent_func(void* arg); // 客服
int main() {
pthread_t smoker1, smoker2, smoker3, a;
sem_init(&tobacco, 0, 0);
sem_init(&paper, 0, 0);
sem_init(&matches, 0, 0);
sem_init(&agent, 0, 1);
if (pthread_create(&smoker1, NULL, smoker_tobacco, NULL) != 0) {
perror("pthread_create error");
exit(1);
}
if (pthread_create(&smoker2, NULL, smoker_paper, NULL) != 0) {
perror("pthread_create error");
exit(1);
}
if (pthread_create(&smoker3, NULL, smoker_matches, NULL) != 0) {
perror("pthread_create error");
exit(1);
}
if (pthread_create(&a, NULL, agent_func, NULL) != 0) {
perror("pthread_create error");
exit(1);
}
pthread_join(smoker1, NULL);
pthread_join(smoker2, NULL);
pthread_join(smoker3, NULL);
pthread_join(a, NULL);
sem_destroy(&tobacco);
sem_destroy(&paper);
sem_destroy(&matches);
sem_destroy(&agent);
return 0;
}
void* smoker_tobacco(void* arg) {
while (1) {
sem_wait(&tobacco);
printf("Smoker1 got tobacco.\n");
sleep(1); // 吸烟
sem_post(&agent);
}
}
void* smoker_paper(void* arg) {
while (1) {
sem_wait(&paper);
printf("Smoker2 got paper.\n");
sleep(1); // 吸烟
sem_post(&agent);
}
}
void* smoker_matches(void* arg) {
while (1) {
sem_wait(&matches);
printf("Smoker3 got matches.\n");
sleep(1); // 吸烟
sem_post(&agent);
}
}
void* agent_func(void* arg) {
int item;
while (1) {
sem_wait(&agent);
item = rand() % 3;
switch (item) {
case 0: // 提供烟草和纸
printf("Agent put tobacco and paper on the table.\n");
sem_post(&matches);
break;
case 1: // 提供烟草和火柴
printf("Agent put tobacco and matches on the table.\n");
sem_post(&paper);
break;
case 2: // 提供纸和火柴
printf("Agent put paper and matches on the table.\n");
sem_post(&tobacco);
break;
}
}
}
```
这个程序中,使用了4个信号量:
- tobacco、paper、matches:表示烟草、纸、火柴的可用状态,初值均为0。
- agent:表示客服的可用状态,初值为1。
吸烟者和客服都是一个无限循环的线程。当客服(agent)发现桌子上有两种需要的材料时,就放开一个信号量,使得对应的吸烟者线程可以获得需要的材料,然后进行吸烟。吸烟者吸烟完毕后,又放开了一个信号量,使得客服线程可以开始下一轮提供材料。
相关推荐
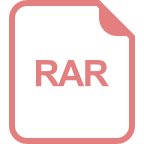














