C++实现:不必定义类,构造升序的单链表并实现逆置
时间: 2024-09-25 09:11:10 浏览: 45
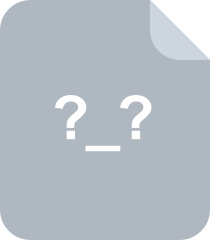
c++代码实现单链表逆置输出
在C++中,我们通常会使用结构体或类来表示链表节点,但如果不需要定义完整的类,可以直接使用裸指针来创建一个简单的单链表。以下是构造升序链表以及逆置链表的示例:
```cpp
#include <iostream>
// 单链表节点定义,这里仅包含数据和指向下一个节点的指针
struct ListNode {
int val;
ListNode* next;
};
// 插入节点,保持升序顺序
void insertSorted(ListNode** head, int val) {
ListNode* node = new ListNode{val, nullptr};
if (*head == nullptr || (*head)->val > val) {
*head = node;
} else {
ListNode* current = *head;
while (current->next && current->next->val < val) {
current = current->next;
}
node->next = current->next;
current->next = node;
}
}
// 逆置链表
ListNode* reverseList(ListNode* head) {
ListNode* prev = nullptr;
ListNode* curr = head;
ListNode* next = nullptr;
while (curr != nullptr) {
next = curr->next;
curr->next = prev;
prev = curr;
curr = next;
}
return prev; // 返回新的头节点
}
int main() {
ListNode* head = nullptr;
// 构造升序链表示例
insertSorted(&head, 5); // 5 -> None
insertSorted(&head, 2); // 2 -> 5 -> None
insertSorted(&head, 8); // 2 -> 5 -> 8 -> None
std::cout << "Original list: ";
printList(head);
head = reverseList(head);
std::cout << "\nReversed list: ";
printList(head);
return 0;
}
// 辅助函数:打印链表
void printList(ListNode* head) {
while (head != nullptr) {
std::cout << head->val << " -> ";
head = head->next;
}
std::cout << "None" << std::endl;
}
```
在这个例子中,`insertSorted` 函数负责按升序插入新节点,而 `reverseList` 函数则通过迭代将链表元素前后交换位置实现逆置。
阅读全文
相关推荐
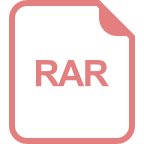
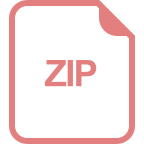















