R语言 使用train函数对随机森林回归预测模型进行十折交叉验证与超参数寻优后,如何计算变量重要性?
时间: 2024-10-11 16:11:02 浏览: 88
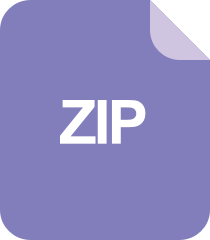
dec-tree-random-forest-titanic:用决策树和随机森林模型预测泰坦尼克号乘客的存活率
在R语言中,使用`caret`包中的`train()`函数训练随机森林回归模型后,通常会通过`varImpPlot()`或`importance()`函数来评估变量的重要性。这里是一个基本步骤:
1. 首先,你需要加载必要的库并导入数据集:
```R
library(caret)
data("your_data_set") # 替换为你的数据集名称
```
2. 使用`train()`进行交叉验证并训练随机森林模型:
```R
set.seed(123) # 设置随机种子以保证结果可重复
rf_model <- train(target_variable ~ ., data = your_data_set, method = "rf", trControl = trainControl(method = "cv", number = 10)) # 可能需要调整参数如ntree、mtry等
```
3. 计算变量重要性:
- 直接从训练好的模型获取变量重要性,可以使用`varImpPlot(rf_model)`,这将生成一个图表显示各特征的重要性。
- 或者,你可以直接查看变量的重要性数值,使用`importance(rf_model)$.importance`,它会返回一个数据框,列名为变量名,行表示重要性。
```R
var_importance <- importance(rf_model)
head(var_importance[, c("variable", "importance")]) # 查看前几项重要性最高的变量
```
阅读全文
相关推荐
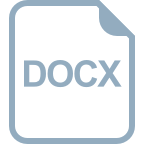
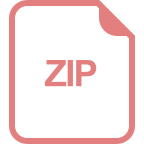




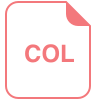
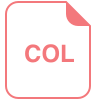
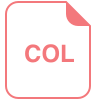
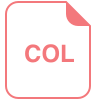
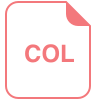
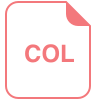
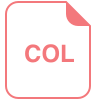
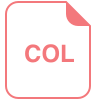
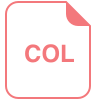
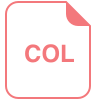
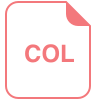
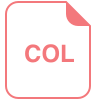