有尾指针的单循环链表
时间: 2024-06-18 14:04:36 浏览: 9
有尾指针的单循环链表是一种特殊的单循环链表,它与普通的单循环链表的区别在于,它的尾节点指针指向了链表的头节点,这样就可以方便地进行链表的插入、删除等操作。
相比于普通单循环链表,有尾指针的单循环链表的插入和删除操作更加方便。例如,在普通单循环链表中,如果要在表尾插入一个节点,则需要遍历整个链表找到尾节点,然后再进行插入操作。而在有尾指针的单循环链表中,只需要直接通过尾节点的指针进行插入即可。
下面是一个示例代码,展示如何实现有尾指针的单循环链表:
```c++
#include <iostream>
using namespace std;
// 定义链表节点结构体
struct Node {
int data;
Node *next;
};
// 定义有尾指针的单循环链表结构体
struct CircleList {
Node *head; // 链表头节点
Node *tail; // 链表尾节点
int size; // 链表大小
// 初始化链表
CircleList() {
head = tail = nullptr;
size = 0;
}
// 在链表尾部插入节点
void insert(int value) {
Node *node = new Node();
node->data = value;
node->next = nullptr;
if (head == nullptr) {
head = tail = node;
} else {
tail->next = node;
tail = node;
}
// 将尾节点指向头节点,形成循环
tail->next = head;
size++;
}
// 删除指定值的节点
void remove(int value) {
Node *prev = nullptr;
Node *curr = head;
while (curr != tail) {
if (curr->data == value) {
break;
}
prev = curr;
curr = curr->next;
}
if (curr->data == value) {
if (prev == nullptr) {
head = curr->next;
tail->next = head;
} else {
prev->next = curr->next;
}
delete curr;
size--;
}
}
// 打印链表
void print() {
Node *curr = head;
while (curr != tail) {
cout << curr->data << " ";
curr = curr->next;
}
cout << curr->data << endl; // 输出尾节点的值
}
};
int main() {
CircleList list;
list.insert(1);
list.insert(2);
list.insert(3);
list.print(); // 输出:1 2 3
list.remove(2);
list.print(); // 输出:1 3
return 0;
}
```
相关推荐
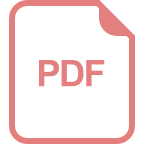
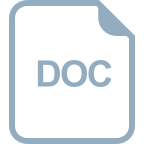
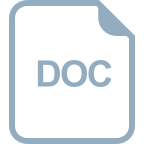














