用jupyter实现以下功能:1.色彩通道转换2.确定颜色区间3.根据颜色区间计算掩模4.将掩模与原始图像做与运算,或根据掩模计算轮廓5.绘制矩形
时间: 2024-10-09 15:09:56 浏览: 66
在Jupyter Notebook中实现这些功能通常会涉及到PIL(Python Imaging Library)或其更新版Pillow库、NumPy以及OpenCV等图像处理库。以下是一些基本步骤:
1. **色彩通道转换**:
- 导入必要的库:`from PIL import Image` 和 `import numpy as np`
```python
image = Image.open('image.jpg')
r, g, b = image.split() # 分离RGB通道
```
2. **确定颜色区间**:
- 使用numpy处理颜色阈值:例如,如果想获取所有红色区域,可以创建一个条件筛选数组
```python
lower_red = (0, 0, 128) # 红色的HSV范围
upper_red = (255, 255, 255)
mask = np.where((r > lower_red[0]) & (r <= upper_red[0]) &
(g > lower_red[1]) & (g <= upper_red[1]) &
(b > lower_red[2]) & (b <= upper_red[2]), 255, 0).astype(np.uint8)
```
3. **计算掩模并与原始图像操作**:
- 使用掩模与原图做位与运算(与运算)
```python
result = np.bitwise_and(image.convert("RGBA"), np.array(mask)[:,:,np.newaxis])
```
4. **根据掩模计算轮廓**:
- 如果需要轮廓,可以先二值化,然后使用OpenCV的findContours函数
```python
_, contours, _ = cv2.findContours(mask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
```
5. **绘制矩形**:
- 根据找到的轮廓,用cv2.drawContours画出矩形
```python
for contour in contours:
x,y,w,h = cv2.boundingRect(contour)
cv2.rectangle(result, (x,y), (x+w, y+h), (0,255,0), 2)
```
记得替换上述代码中的'image.jpg'为你实际要处理的图片路径。
阅读全文
相关推荐
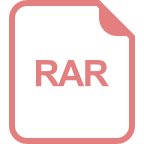
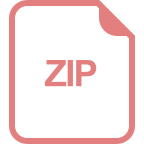


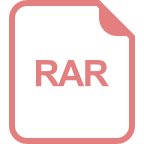
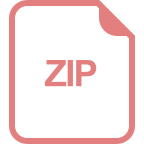
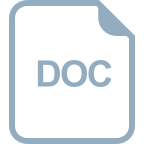
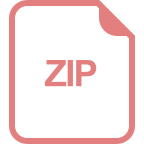
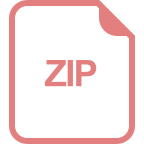
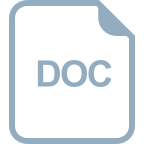
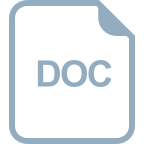
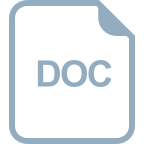





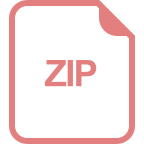
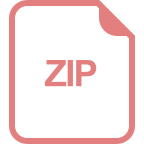