16、输入两个整数x,y输出x,y中各位数字重新组合可能形成的最大的数输入:只有一组,每组两个长度不超12位的正整数输出:用x,y中的所有的数字位重新组合能形成的最大的数样例输入:123456样例输出654321 使用C语言
时间: 2024-12-06 21:23:05 浏览: 21
为了实现这个功能,你可以使用C语言编写一个程序,包括以下几个步骤:
1. **读取输入**:
首先从标准输入读取两个12位以内的正整数x和y。
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
int x, y;
scanf("%d %d", &x, &y);
}
```
2. **分离数字**:
分别提取x和y的每一位数字并存储在一个数组中。
```c
char* numArray[2][13];
int index = 0;
while (x > 0) {
numArray[0][index++] = (x % 10) + '0';
x /= 10;
}
while (y > 0) {
numArray[1][index++] = (y % 10) + '0';
y /= 10;
}
```
3. **构造最大数**:
初始化一个大整数结构,然后按照降序将数字添加到字符串中。
```c
long long result = 0;
for (int i = index - 1; i >= 0; i--) {
result = (result * 10) + atoi(numArray[0] + i);
result = (result * 10) + atoi(numArray[1] + i);
}
```
4. **输出结果**:
最后转换结果为字符串并输出。
```c
char output[25];
sprintf(output, "%lld", result);
printf("%s\n", output);
```
完整的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 将字符数组转换为整数
int atoi(char* str) {
long long value = 0;
while (*str != '\0') {
value *= 10;
value += *str - '0';
str++;
}
return value;
}
int main() {
int x, y;
scanf("%d %d", &x, &y);
char* numArray[2][13];
int index = 0;
while (x > 0) {
numArray[0][index++] = (x % 10) + '0';
x /= 10;
}
while (y > 0) {
numArray[1][index++] = (y % 10) + '0';
y /= 10;
}
long long result = 0;
for (int i = index - 1; i >= 0; i--) {
result = (result * 10) + atoi(numArray[0] + i);
result = (result * 10) + atoi(numArray[1] + i);
}
char output[25];
sprintf(output, "%lld", result);
printf("%s\n", output);
return 0;
}
```
阅读全文
相关推荐
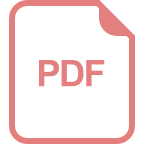
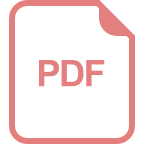
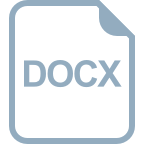
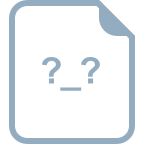
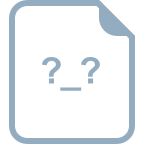
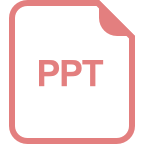
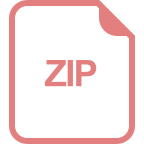
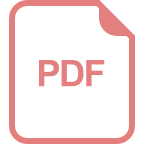
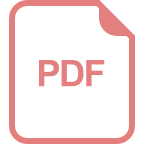
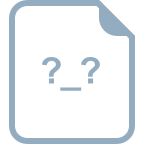
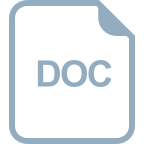
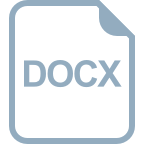
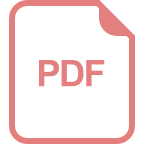
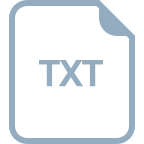
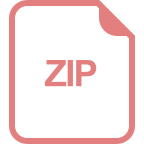
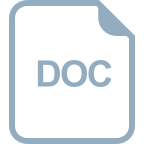
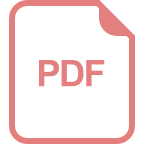
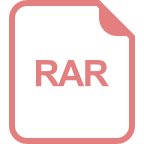