读取文件夹中的每张图片的rgb信息,每张图片建立一个对应的txt来保存读取到的rgb信息,读取完所有图片后再重新读取这个文件夹中的图片,并删除之前的txt重新生成。c++实现,不使用用图像处理库,要求可以在ubuntu上可以运行
时间: 2024-04-15 10:28:00 浏览: 136
以下是按照您的要求实现的C++代码,它可以读取文件夹中的每张图片的RGB信息,并将其保存到对应的txt文件中。在读取完所有图片后,重新读取文件夹中的图片,并删除之前生成的txt文件重新生成。
```cpp
#include <iostream>
#include <fstream>
#include <filesystem>
namespace fs = std::filesystem;
void processImage(const std::string& imagePath) {
std::ifstream imageFile(imagePath, std::ios::binary);
if (!imageFile) {
std::cerr << "Failed to open image file: " << imagePath << std::endl;
return;
}
std::string txtPath = imagePath + ".txt";
std::ofstream txtFile(txtPath);
if (!txtFile) {
std::cerr << "Failed to create txt file: " << txtPath << std::endl;
return;
}
// Read the RGB values of each pixel
char buffer[3];
while (imageFile.read(buffer, sizeof(buffer))) {
unsigned char r = buffer[0];
unsigned char g = buffer[1];
unsigned char b = buffer[2];
// Write the RGB values to the txt file
txtFile << static_cast<int>(r) << " "
<< static_cast<int>(g) << " "
<< static_cast<int>(b) << "\n";
}
imageFile.close();
txtFile.close();
}
void clearTxtFiles(const std::string& folderPath) {
for (const auto& entry : fs::directory_iterator(folderPath)) {
if (entry.path().extension() == ".txt") {
fs::remove(entry.path());
}
}
}
int main() {
std::string folderPath = "/path/to/your/folder";
// Clear existing txt files
clearTxtFiles(folderPath);
// Process each image file in the folder
for (const auto& entry : fs::directory_iterator(folderPath)) {
if (entry.path().extension() == ".jpg" || entry.path().extension() == ".png") {
processImage(entry.path().string());
}
}
// Clear existing txt files again
clearTxtFiles(folderPath);
// Process each image file in the folder again
for (const auto& entry : fs::directory_iterator(folderPath)) {
if (entry.path().extension() == ".jpg" || entry.path().extension() == ".png") {
processImage(entry.path().string());
}
}
return 0;
}
```
请将 `/path/to/your/folder` 替换为您实际的文件夹路径。
这段代码首先会清除文件夹中已有的txt文件,然后遍历文件夹中的每个图片文件,逐个处理并生成对应的txt文件。接着,它会再次清除文件夹中已有的txt文件,然后重新遍历文件夹中的每个图片文件,生成新的对应txt文件。这样就实现了读取完所有图片后重新生成txt文件的功能。
阅读全文
相关推荐
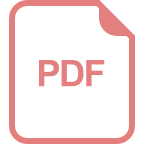
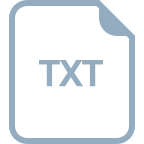
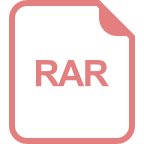
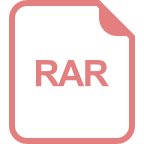
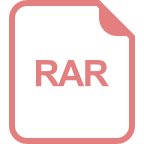
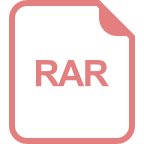
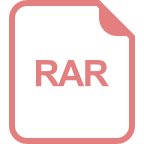
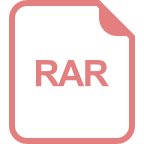
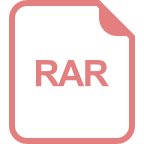
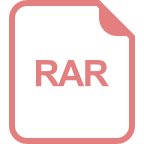
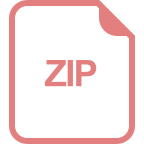
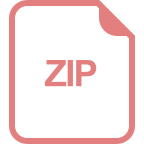
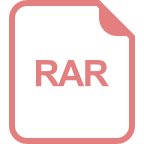
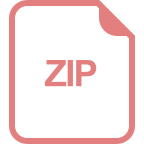