在springboot项目中连接sql server的mapper怎么写?
时间: 2023-08-31 17:31:39 浏览: 324
### 回答1:
在 Spring Boot 项目中连接 SQL Server 的 mapper 可以通过使用 MyBatis 或者 JPA 等框架来实现。
MyBatis 的配置步骤如下:
1. 在项目中引入 MyBatis 的依赖。
2. 配置数据源。
3. 在 mapper 接口中声明映射的 SQL 语句。
4. 在对应的 XML 文件中编写 SQL 语句。
5. 在 Application 类中配置 MyBatis。
JPA 的配置步骤如下:
1. 在项目中引入 JPA 的依赖。
2. 配置数据源。
3. 创建 Entity 类,用于映射数据库中的表。
4. 创建 Repository 接口,用于操作数据库。
5. 在 Application 类中启用 JPA。
请注意,上述配置步骤仅是大致步骤,具体的配置方式可以参考官方文档。
### 回答2:
在Spring Boot项目中连接SQL Server时,需要进行一些配置和编写相关的Mapper。
首先,添加SQL Server依赖项。在pom.xml文件中,添加以下依赖项:
```xml
<dependency>
<groupId>com.microsoft.sqlserver</groupId>
<artifactId>mssql-jdbc</artifactId>
<version>7.4.1.jre11</version>
</dependency>
```
接下来,在application.properties文件中配置数据源信息:
```properties
spring.datasource.url=jdbc:sqlserver://localhost:1433;databaseName=mydatabase
spring.datasource.driver-class-name=com.microsoft.sqlserver.jdbc.SQLServerDriver
spring.datasource.username=sa
spring.datasource.password=your_password
```
请将上述配置中的URL、用户名和密码替换为真实的SQL Server连接信息。
然后,创建一个Mapper接口,用于书写对数据库的操作方法。例如,我们创建一个UserMapper接口:
```java
@Repository
public interface UserMapper {
@Select("SELECT * FROM users WHERE id = #{id}")
User getUserById(Integer id);
@Insert("INSERT INTO users (name, age) VALUES (#{name}, #{age})")
@Options(useGeneratedKeys = true, keyProperty = "id")
void insertUser(User user);
@Update("UPDATE users SET name = #{name}, age = #{age} WHERE id = #{id}")
void updateUser(User user);
@Delete("DELETE FROM users WHERE id = #{id}")
void deleteUser(Integer id);
}
```
在上述示例中,@Select、@Insert、@Update和@Delete是MyBatis框架提供的注解,用于执行SQL语句。其中,@Options注解用于生成自增的主键值。
最后,通过在Service文件中注入UserMapper,并调用其方法来实现对数据库的访问。
以上是在Spring Boot项目中连接SQL Server的Mapper的编写方法。根据实际情况,需要根据数据库表结构和需求编写相应的SQL语句。
### 回答3:
在Spring Boot项目中连接SQL Server的mapper可以按照以下步骤进行编写。
首先,确保你的Spring Boot项目已经添加了SQL Server的依赖,例如在pom.xml文件中加入以下依赖:
```
<dependency>
<groupId>com.microsoft.sqlserver</groupId>
<artifactId>mssql-jdbc</artifactId>
</dependency>
```
接下来,在application.properties(或application.yml)文件中配置SQL Server的连接信息,例如:
```
spring.datasource.url=jdbc:sqlserver://localhost:1433;databaseName=your_database_name
spring.datasource.username=your_username
spring.datasource.password=your_password
spring.datasource.driver-class-name=com.microsoft.sqlserver.jdbc.SQLServerDriver
```
然后,创建一个数据访问接口(通常以Mapper结尾),并在接口中定义需要的数据操作方法。例如:
```java
@Repository
public interface YourMapper {
@Select("SELECT * FROM your_table")
List<YourEntity> getAllData();
}
```
注意:需要添加`@Repository`注解来声明这是一个Spring组件。
最后,你可以在需要使用Mapper的地方通过依赖注入的方式来使用该Mapper。例如,在Service层或Controller层:
```java
@Service
public class YourService {
private final YourMapper yourMapper;
public YourService(YourMapper yourMapper) {
this.yourMapper = yourMapper;
}
public List<YourEntity> getAllData() {
return yourMapper.getAllData();
}
}
```
这样,你就可以在Spring Boot项目中连接SQL Server并通过Mapper进行数据访问了。当然,如果有其他特殊的需求,你还可以在Mapper接口中定义更多的数据操作方法。
阅读全文
相关推荐
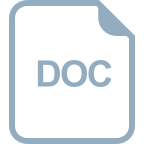
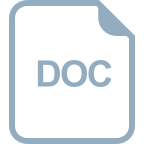
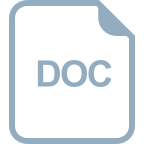
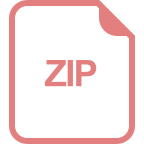
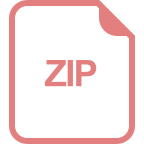
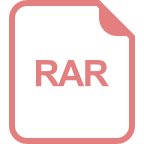
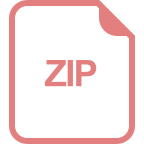






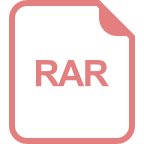
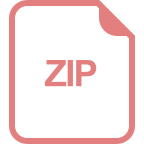



