用python实现完整维吉尼亚演示程序并形成可视化界面
时间: 2024-01-22 18:18:17 浏览: 23
要实现维吉尼亚加密算法的完整演示程序,并形成可视化界面,可以采用以下步骤:
1. 实现维吉尼亚加密算法的加密和解密函数。
2. 编写能够读取用户输入的程序界面,包括输入明文、密钥和选择加密/解密等选项。
3. 在程序界面中添加加密和解密按钮,点击按钮后调用对应的加密/解密函数。
4. 将加密后的密文输出到程序界面中。
下面是一个使用 Python tkinter 库实现维吉尼亚加密算法的演示程序的示例代码:
```python
import tkinter as tk
def encrypt(plaintext, key):
# 实现维吉尼亚加密算法的加密函数
ciphertext = ''
key_len = len(key)
for i, c in enumerate(plaintext):
shift = ord(key[i % key_len]) - 97
shifted_c = chr((ord(c) - 97 + shift) % 26 + 97)
ciphertext += shifted_c
return ciphertext
def decrypt(ciphertext, key):
# 实现维吉尼亚加密算法的解密函数
plaintext = ''
key_len = len(key)
for i, c in enumerate(ciphertext):
shift = ord(key[i % key_len]) - 97
shifted_c = chr((ord(c) - 97 - shift) % 26 + 97)
plaintext += shifted_c
return plaintext
def encrypt_text():
# 点击加密按钮时调用的函数
plaintext = plaintext_input.get()
key = key_input.get()
ciphertext = encrypt(plaintext, key)
ciphertext_output.delete('1.0', tk.END)
ciphertext_output.insert('1.0', ciphertext)
def decrypt_text():
# 点击解密按钮时调用的函数
ciphertext = ciphertext_input.get()
key = key_input.get()
plaintext = decrypt(ciphertext, key)
plaintext_output.delete('1.0', tk.END)
plaintext_output.insert('1.0', plaintext)
# 创建程序界面
root = tk.Tk()
root.title('维吉尼亚加密算法演示程序')
# 明文输入框
plaintext_label = tk.Label(root, text='明文')
plaintext_label.grid(row=0, column=0)
plaintext_input = tk.Entry(root)
plaintext_input.grid(row=0, column=1)
# 密文输入框
ciphertext_label = tk.Label(root, text='密文')
ciphertext_label.grid(row=1, column=0)
ciphertext_input = tk.Entry(root)
ciphertext_input.grid(row=1, column=1)
# 密钥输入框
key_label = tk.Label(root, text='密钥')
key_label.grid(row=2, column=0)
key_input = tk.Entry(root)
key_input.grid(row=2, column=1)
# 加密按钮
encrypt_button = tk.Button(root, text='加密', command=encrypt_text)
encrypt_button.grid(row=3, column=0)
# 解密按钮
decrypt_button = tk.Button(root, text='解密', command=decrypt_text)
decrypt_button.grid(row=3, column=1)
# 明文输出框
plaintext_output_label = tk.Label(root, text='明文')
plaintext_output_label.grid(row=4, column=0)
plaintext_output = tk.Text(root, height=5, width=30)
plaintext_output.grid(row=5, column=0)
# 密文输出框
ciphertext_output_label = tk.Label(root, text='密文')
ciphertext_output_label.grid(row=4, column=1)
ciphertext_output = tk.Text(root, height=5, width=30)
ciphertext_output.grid(row=5, column=1)
root.mainloop()
```
可以看到,这个程序界面包括了明文、密文和密钥的输入框,以及加密和解密按钮。在用户输入明文、密钥和选择加密/解密等选项后,点击加密/解密按钮时会调用对应的加密/解密函数,并将结果输出到程序界面中。
相关推荐
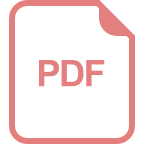
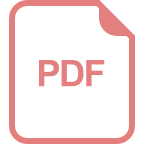
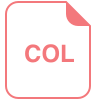

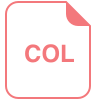
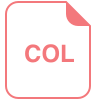
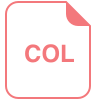









