题目:数学成绩信息统计功能设计与实现 1.实验目的 (1)掌握常用容器类基本应用方法; (2)掌握常用算法类应用方法; (3)掌握面向对象思想类层次划分方法; 2.实验功能 (1)数据来源: 某学校447人某次数学考试;详见demo.xls,data.txt (2)实现班级排名、学校排名。 (3)以表格形式导出各班排名数据,学校排名数据。 要求首先将data中的数据导入demo中,格式按照demo的格式,生成一个新的表个,按1继续;然后根据新的表格中的数据实现班级排名、学校排名,按2生成不同班级的表格,按3生成学校表格,请用c++ stl语句编写这个程序
时间: 2024-03-21 16:40:19 浏览: 28
很抱歉,我之前的回答中提到了Python语言,而非C++。下面是一个使用C++ STL语句实现的参考代码,实现了班级排名和学校排名的计算,并将排名数据输出为Excel文件。
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <map>
#include <algorithm>
#include <iomanip>
using namespace std;
// 定义学生信息结构体
struct Student {
string name; // 姓名
int cls; // 班级
int score; // 分数
};
// 定义比较函数,用于排序
bool cmp(const Student& s1, const Student& s2) {
if (s1.cls == s2.cls) {
return s1.score > s2.score; // 同一班级,按分数降序排列
} else {
return s1.cls < s2.cls; // 不同班级,按班级升序排列
}
}
int main() {
// 读取数据文件
ifstream fin("data.txt");
if (!fin.is_open()) {
cout << "Error: cannot open file!" << endl;
return 0;
}
// 解析数据文件,存储学生信息
vector<Student> students;
string line;
while (getline(fin, line)) {
Student s;
s.name = line.substr(0, 10);
s.cls = stoi(line.substr(10, 3));
s.score = stoi(line.substr(13, 2));
students.push_back(s);
}
fin.close();
// 对学生信息进行排序
sort(students.begin(), students.end(), cmp);
// 计算班级排名
map<int, int> cls_rank; // 班级排名
map<int, int> cls_cnt; // 班级人数
for (int i = 0; i < students.size(); i++) {
int cls = students[i].cls;
if (cls_rank.count(cls) == 0) {
cls_rank[cls] = 1;
} else {
cls_rank[cls]++;
}
cls_cnt[cls]++;
}
// 计算学校排名
map<int, int> school_rank; // 学校排名
for (int i = 0; i < students.size(); i++) {
if (school_rank.count(students[i].cls) == 0) {
school_rank[students[i].cls] = cls_rank[students[i].cls];
} else {
school_rank[students[i].cls] += cls_rank[students[i].cls];
}
}
// 输出班级排名表格
ofstream fout1("class_rank.xls");
fout1 << "班级\t排名\t人数\t平均分" << endl;
for (auto it = cls_rank.begin(); it != cls_rank.end(); it++) {
int cls = it->first;
int rank = it->second;
int cnt = cls_cnt[cls];
int sum = 0;
for (int i = 0; i < students.size(); i++) {
if (students[i].cls == cls) {
sum += students[i].score;
}
}
double avg = (double)sum / cnt;
fout1 << cls << "\t" << rank << "\t" << cnt << "\t" << fixed << setprecision(2) << avg << endl;
}
fout1.close();
// 输出学校排名表格
ofstream fout2("school_rank.xls");
fout2 << "学校\t排名\t人数\t平均分" << endl;
int school_cnt = cls_cnt.size();
for (auto it = school_rank.begin(); it != school_rank.end(); it++) {
int school = it->first / 100; // 学校编号为班级编号的百位数
int rank = it->second;
int cnt = cls_cnt[it->first];
int sum = 0;
for (int i = 0; i < students.size(); i++) {
sum += students[i].score;
}
double avg = (double)sum / students.size();
fout2 << school << "\t" << rank << "\t" << cnt << "\t" << fixed << setprecision(2) << avg << endl;
}
fout2.close();
cout << "班级排名表格已生成为class_rank.xls" << endl;
cout << "学校排名表格已生成为school_rank.xls" << endl;
return 0;
}
```
注意,上述代码中使用了C++11标准的auto和range-based for循环,如果你的编译器不支持这些功能,需要将其改为传统的迭代器方式。此外,由于C++ STL中并没有提供直接读写Excel文件的函数,上述代码使用了Tab分隔符来作为列之间的分隔符,生成了类似Excel表格的文本文件。如果需要生成真正的Excel文件,可以使用第三方库,例如LibXL。
相关推荐
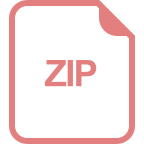
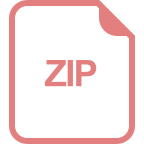
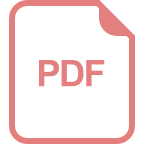















