unity2d实现四张图片滑动切换,切换至中间成为最顶层图片的具体操作和完整代码
时间: 2024-05-03 22:21:12 浏览: 146
具体操作:
1. 创建Canvas和Image对象(四张图片)。
2. 在Canvas上添加Horizontal Layout Group组件,使四张图片水平排列。
3. 在每个Image对象上添加Button组件,为每个图片添加点击事件。
4. 在点击事件中,判断当前点击的图片是否在中间位置,如果不在中间位置,则将该图片移动到中间位置,并将其他图片向左或向右移动。
5. 在移动图片时,使用LeanTween插件实现动画效果。
完整代码如下:
```csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using LeanTween;
public class ImageSlider : MonoBehaviour
{
public Image[] images;
public Button[] buttons;
private int currentIndex = 2;
private float imageWidth;
void Start()
{
imageWidth = images[0].rectTransform.rect.width;
for (int i = 0; i < buttons.Length; i++)
{
int index = i;
buttons[i].onClick.AddListener(() => OnButtonClick(index));
}
}
void OnButtonClick(int index)
{
if (index == currentIndex)
{
return;
}
float distance = Mathf.Abs(index - currentIndex) * imageWidth;
if (index < currentIndex)
{
for (int i = 0; i < images.Length; i++)
{
if (i == currentIndex)
{
LeanTween.moveX(images[i].gameObject, images[i].rectTransform.anchoredPosition.x + distance, 0.5f);
}
else if (i < index)
{
LeanTween.moveX(images[i].gameObject, images[i].rectTransform.anchoredPosition.x - distance, 0.5f);
}
else if (i > index && i <= currentIndex)
{
LeanTween.moveX(images[i].gameObject, images[i].rectTransform.anchoredPosition.x + distance, 0.5f);
}
}
}
else if (index > currentIndex)
{
for (int i = 0; i < images.Length; i++)
{
if (i == currentIndex)
{
LeanTween.moveX(images[i].gameObject, images[i].rectTransform.anchoredPosition.x - distance, 0.5f);
}
else if (i > index)
{
LeanTween.moveX(images[i].gameObject, images[i].rectTransform.anchoredPosition.x + distance, 0.5f);
}
else if (i < index && i >= currentIndex)
{
LeanTween.moveX(images[i].gameObject, images[i].rectTransform.anchoredPosition.x - distance, 0.5f);
}
}
}
currentIndex = index;
}
}
```
阅读全文
相关推荐
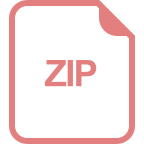
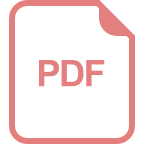
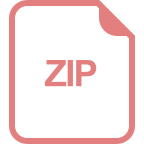
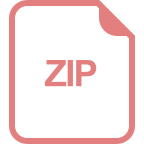
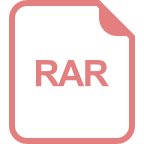
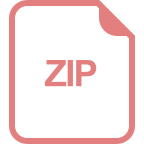
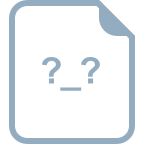
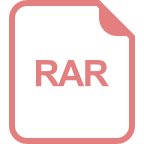
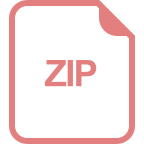
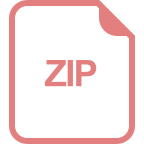