python监控网页变化
时间: 2023-08-11 15:03:15 浏览: 348
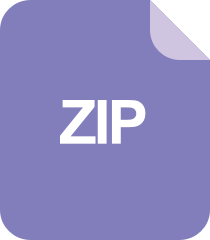
python一款监控端口变化的系统
要监控网页变化,可以使用 Python 的第三方库 BeautifulSoup 和 requests。具体步骤如下:
1. 使用 requests 库获取网页 HTML 内容;
2. 使用 BeautifulSoup 库解析 HTML 内容;
3. 通过定时器不断重复上述步骤,比较两次解析结果是否一致,若不一致,则说明网页发生了变化。
以下是一个简单的示例代码:
```python
import requests
from bs4 import BeautifulSoup
import time
url = 'https://www.example.com'
last_content = ''
while True:
# 发送 GET 请求,获取 HTML 内容
response = requests.get(url)
content = response.content.decode('utf-8')
# 解析 HTML 内容
soup = BeautifulSoup(content, 'html.parser')
# 比较两次解析结果是否一致
if content != last_content:
print('网页发生了变化!')
# 更新上一次的解析结果
last_content = content
# 暂停 1 秒钟再次请求
time.sleep(1)
```
记得替换 `url` 变量为你想要监控的网页地址。
阅读全文
相关推荐
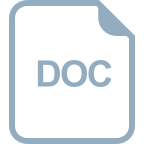



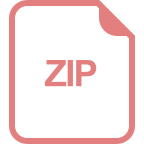
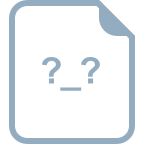
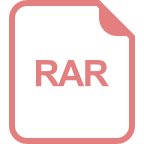
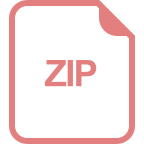
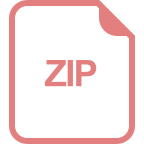
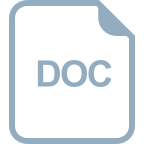
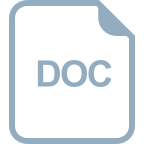
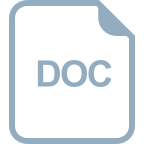
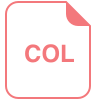
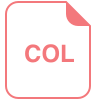
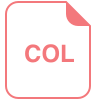