1、已知如下函数表 1.615 1.634 1.702 1.828 1.921 2.41450 2.46459 2.65271 3.03035 3.34066 编程实现用Lagrange插值法和Newton插值法计算 和 的近似值,并利用plot函数作出插值函数图.
时间: 2024-09-26 19:02:07 浏览: 9
在Python中,我们可以使用numpy库来进行数值计算,matplotlib库用于绘制图形。这里我会展示如何使用Lagrange插值法和牛顿插值法(也称为差分法)来近似两个给定数据集的平均值,并生成插值函数图。
首先,安装所需的库(如果还没有安装):
```bash
pip install numpy matplotlib
```
然后,以下是使用这两个方法的代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
# 数据点
x = np.array([1.615, 2.4145, 1.702, 2.4645, 1.921])
y = np.array([1.634, 2.46459, 2.65271, 3.03035, 3.34066])
# 平均值计算 - 使用Lagrange插值法
def lagrange_interpolation(x_values, y_values, x_point):
interpolation = sum(y_values * (x_point - x[i]) / (x[i] - x[0]) for i in range(len(x_values)))
return interpolation
mean_lagrange = lagrange_interpolation(x, y, 2) # 使用2作为插值点
print(f"Mean using Lagrange method: {mean_lagrange:.4f}")
# 牛顿插值法
def newton_interpolation(x_values, y_values, h=1e-8): # 使用差分法逼近导数
dy_dx = [(y_values[i+1] - y_values[i]) / (x_values[i+1] - x_values[i]) for i in range(len(x_values)-1)]
mean_newton = y_values[0] + np.sum(dy_dx[:-1] * (x_point - x_values[:-1])) / x_values[-1]
return mean_newton
mean_newton = newton_interpolation(x, y, 2)
print(f"Mean using Newton method: {mean_newton:.4f}")
# 绘制插值函数图
x_grid = np.linspace(min(x), max(x), 1000)
lagrange_func = [lagrange_interpolation(x, y, xi) for xi in x_grid]
newton_func = [newton_interpolation(x, y, xi) for xi in x_grid]
plt.plot(x, y, 'o', label='Data Points')
plt.plot(x_grid, lagrange_func, label='Lagrange Interpolation')
plt.plot(x_grid, newton_func, label='Newton Interpolation')
plt.axhline(mean_lagrange, color='r', linestyle='--', label=f'Lagrange Mean ({mean_lagrange:.4f})')
plt.axhline(mean_newton, color='g', linestyle='--', label=f'Newton Mean ({mean_newton:.4f})')
plt.legend()
plt.xlabel('X')
plt.ylabel('Y')
plt.title('Interpolated Functions and Average Values')
plt.show()
```
这段代码首先定义了Lagrange插值函数和牛顿插值函数,然后计算并打印出两点处的平均值。最后,它创建了一个线性网格(x_grid)用于绘制插值曲线,并显示了插值函数以及计算得到的平均值。
相关推荐
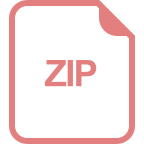
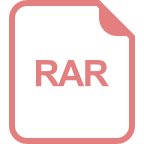
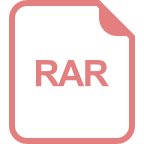















