详细介绍C语言中的数学函数库,介绍里面的各个函数的具体用法并给出代码示例
时间: 2023-08-28 10:05:09 浏览: 54
C语言中的数学函数库是math.h,它提供了许多常用的数学函数,如三角函数、指数函数、对数函数、幂函数、取整函数等等。
下面是math.h中一些常用的函数及其用法:
1. sin(x):计算正弦函数的值,x为弧度制的角度。
```c
#include <math.h>
#include <stdio.h>
int main()
{
double angle = 30.0;
double radian = angle * M_PI / 180.0;
double result = sin(radian);
printf("sin(%lf) = %lf\n", angle, result);
return 0;
}
```
2. cos(x):计算余弦函数的值,x为弧度制的角度。
```c
#include <math.h>
#include <stdio.h>
int main()
{
double angle = 60.0;
double radian = angle * M_PI / 180.0;
double result = cos(radian);
printf("cos(%lf) = %lf\n", angle, result);
return 0;
}
```
3. tan(x):计算正切函数的值,x为弧度制的角度。
```c
#include <math.h>
#include <stdio.h>
int main()
{
double angle = 45.0;
double radian = angle * M_PI / 180.0;
double result = tan(radian);
printf("tan(%lf) = %lf\n", angle, result);
return 0;
}
```
4. exp(x):计算e的x次方。
```c
#include <math.h>
#include <stdio.h>
int main()
{
double x = 2.0;
double result = exp(x);
printf("e^%lf = %lf\n", x, result);
return 0;
}
```
5. log(x):计算以e为底数的对数。
```c
#include <math.h>
#include <stdio.h>
int main()
{
double x = 10.0;
double result = log(x);
printf("ln(%lf) = %lf\n", x, result);
return 0;
}
```
6. pow(x, y):计算x的y次方。
```c
#include <math.h>
#include <stdio.h>
int main()
{
double x = 2.0;
double y = 3.0;
double result = pow(x, y);
printf("%lf^%lf = %lf\n", x, y, result);
return 0;
}
```
7. ceil(x):对x向上取整。
```c
#include <math.h>
#include <stdio.h>
int main()
{
double x = 2.4;
double result = ceil(x);
printf("ceil(%lf) = %lf\n", x, result);
return 0;
}
```
8. floor(x):对x向下取整。
```c
#include <math.h>
#include <stdio.h>
int main()
{
double x = 2.9;
double result = floor(x);
printf("floor(%lf) = %lf\n", x, result);
return 0;
}
```
以上是math.h中常用函数的一些示例代码,还有许多函数没有列举出来,有兴趣的读者可以自行查阅相关资料。
相关推荐
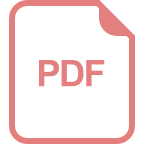
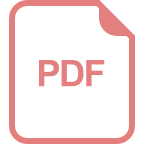
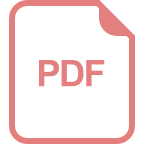
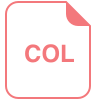
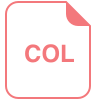
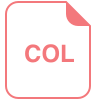
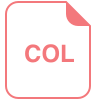
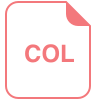









