请选择实现一种网络模型,LeNet、VGG或者ResNet,并在Mnist数据集和Fashion Mnist数据集上进行训练和验证
时间: 2024-02-06 11:11:48 浏览: 39
好的,我选择ResNet网络模型,这是一种非常经典的深度卷积神经网络模型。
首先,我们需要导入必要的库和数据集。以下是代码:
```python
import tensorflow as tf
from tensorflow.keras import layers, models, datasets
# 加载Mnist数据集和Fashion Mnist数据集
(train_images, train_labels), (test_images, test_labels) = datasets.mnist.load_data()
(fashion_train_images, fashion_train_labels), (fashion_test_images, fashion_test_labels) = datasets.fashion_mnist.load_data()
# 将像素值缩放到0到1之间
train_images, test_images = train_images / 255.0, test_images / 255.0
fashion_train_images, fashion_test_images = fashion_train_images / 255.0, fashion_test_images / 255.0
```
接下来,我们可以构建ResNet模型。以下是代码:
```python
def resnet_block(input_data, filters, kernel_size, stride):
x = layers.Conv2D(filters, kernel_size=kernel_size, strides=stride, padding='same', activation='relu')(input_data)
x = layers.BatchNormalization()(x)
x = layers.Conv2D(filters, kernel_size=kernel_size, strides=1, padding='same', activation=None)(x)
x = layers.BatchNormalization()(x)
x = layers.add([x, input_data])
x = layers.Activation('relu')(x)
return x
def resnet(input_shape, num_classes):
inputs = layers.Input(shape=input_shape)
x = layers.Conv2D(64, kernel_size=7, strides=2, padding='same', activation='relu')(inputs)
x = layers.BatchNormalization()(x)
x = layers.MaxPooling2D(pool_size=(3, 3), strides=2, padding='same')(x)
# ResNet blocks
x = resnet_block(x, 64, 3, 1)
x = resnet_block(x, 64, 3, 1)
x = resnet_block(x, 128, 3, 2)
x = resnet_block(x, 128, 3, 1)
x = resnet_block(x, 256, 3, 2)
x = resnet_block(x, 256, 3, 1)
x = layers.GlobalAveragePooling2D()(x)
# 输出层
outputs = layers.Dense(num_classes, activation='softmax')(x)
model = models.Model(inputs=inputs, outputs=outputs)
return model
# 构建模型
model = resnet((28,28,1), 10)
model.summary()
```
在上述代码中,我们定义了一个ResNet块函数和一个ResNet模型函数。在ResNet模型函数中,我们首先定义了模型的输入层,然后添加一个卷积层和一个批量归一化层。接下来,我们添加了一些ResNet块,以增加模型的深度。最后,我们添加了一个全局平均池化层和一个输出层。
现在我们可以编译和训练模型。以下是代码:
```python
# 编译模型
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# 训练模型
history = model.fit(train_images[..., tf.newaxis], train_labels, epochs=10, validation_data=(test_images[..., tf.newaxis], test_labels))
```
在上述代码中,我们使用了Adam优化器和稀疏分类交叉熵损失函数来编译模型。然后,我们使用Mnist数据集来训练模型,并在测试集上进行验证。
我们还可以使用Fashion Mnist数据集来验证模型的泛化能力。以下是代码:
```python
# 在Fashion Mnist数据集上进行测试
fashion_loss, fashion_acc = model.evaluate(fashion_test_images[..., tf.newaxis], fashion_test_labels)
print('Fashion Mnist accuracy:', fashion_acc)
```
最后,我们可以绘制训练和验证的准确率和损失函数图表,以便更好地了解模型的性能。以下是代码:
```python
import matplotlib.pyplot as plt
# 绘制准确率图表
plt.plot(history.history['accuracy'], label='accuracy')
plt.plot(history.history['val_accuracy'], label='val_accuracy')
plt.xlabel('Epoch')
plt.ylabel('Accuracy')
plt.ylim([0.5, 1])
plt.legend(loc='lower right')
plt.show()
# 绘制损失函数图表
plt.plot(history.history['loss'], label='loss')
plt.plot(history.history['val_loss'], label='val_loss')
plt.xlabel('Epoch')
plt.ylabel('Loss')
plt.legend(loc='upper right')
plt.show()
```
这样,我们就成功地在Mnist数据集和Fashion Mnist数据集上使用ResNet模型进行了训练和验证。
相关推荐
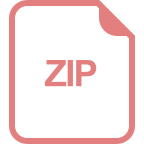
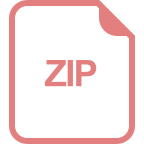
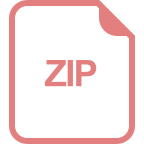














