在Linux下对文件进行监控,并且禁止不满足条件的进程对该文件进行访问,访问该文件的进程来自与其他程序。请你用C/C++写出来,并加上注释
时间: 2024-04-29 16:25:35 浏览: 16
这个问题需要使用Linux下的inotify API和文件访问控制相关的系统调用来实现。
以下是一个简单的示例,可以监控指定文件的访问,并禁止不满足条件的进程对该文件进行访问:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include <unistd.h>
#include <sys/inotify.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#define EVENT_SIZE (sizeof(struct inotify_event))
#define EVENT_BUF_LEN (1024 * (EVENT_SIZE + 16))
int main(int argc, char **argv) {
if (argc != 2) {
printf("Usage: %s <file>\n", argv[0]);
return 1;
}
int fd = inotify_init(); // 初始化inotify实例
if (fd < 0) {
perror("inotify_init");
return 1;
}
int wd = inotify_add_watch(fd, argv[1], IN_OPEN); // 添加监控
if (wd < 0) {
perror("inotify_add_watch");
return 1;
}
char buf[EVENT_BUF_LEN];
while (1) {
int len = read(fd, buf, EVENT_BUF_LEN); // 读取事件
if (len < 0) {
perror("read");
return 1;
}
int i = 0;
while (i < len) {
struct inotify_event *event = (struct inotify_event *) &buf[i];
if (event->mask & IN_OPEN) { // 有进程打开了文件
struct stat st;
if (stat(argv[1], &st) == 0) {
if ((st.st_mode & S_IWOTH) != 0) { // 文件对其他用户可写
printf("Permission denied!\n");
int pid = fork(); // 创建子进程以禁止访问
if (pid == 0) {
execl("/usr/bin/chmod", "chmod", "o-w", argv[1], NULL);
perror("execl");
exit(1);
}
waitpid(pid, NULL, 0);
}
} else {
perror("stat");
}
}
i += EVENT_SIZE + event->len;
}
}
inotify_rm_watch(fd, wd); // 移除监控
close(fd);
return 0;
}
```
程序的基本思路如下:
1. 使用inotify_init函数初始化inotify实例。
2. 使用inotify_add_watch函数添加对指定文件的监控。
3. 循环调用read函数读取事件,处理每个事件。
4. 检查事件是否为文件打开事件(IN_OPEN),如果是则继续处理。
5. 使用stat函数获取文件的权限信息,检查文件是否对其他用户可写(S_IWOTH)。
6. 如果文件对其他用户可写,则禁止访问该文件。
7. 使用fork函数创建子进程,使用execl函数调用chmod命令修改文件权限(去除其他用户的写权限)。
8. 等待子进程结束。
9. 继续循环处理事件。
10. 使用inotify_rm_watch函数移除对文件的监控。
11. 关闭inotify实例。
需要注意的是,这个示例程序只是一个简单的演示,实际应用中还需要考虑更多的细节和安全问题。例如,需要对禁止访问的进程进行鉴定和记录,防止恶意进程绕过访问控制。
相关推荐
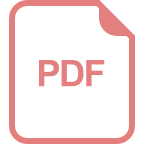
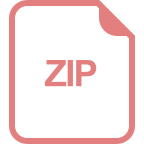
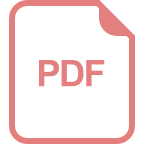
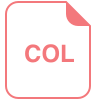
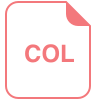
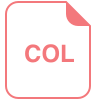
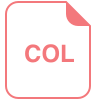
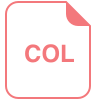









