如何使用 com.itextpdf 5.5.10 导出pdf表格,提供一个pdf导出工具类,并在工具类中提供支持单元格合并的方法
时间: 2024-03-16 07:47:50 浏览: 119
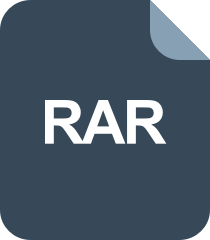
itextpdf按模版导出PDF(表单,表格,条码,二维码)

下面是一个使用 iTextPDF 5.5.10 导出 PDF 表格的示例工具类,其中包含支持单元格合并的方法:
```java
import com.itextpdf.text.Document;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.PageSize;
import com.itextpdf.text.pdf.PdfPCell;
import com.itextpdf.text.pdf.PdfPTable;
import com.itextpdf.text.pdf.PdfWriter;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
public class PDFExporter {
public static void exportTableToPDF(String filePath, String[] header, String[][] data) {
Document document = new Document(PageSize.A4.rotate());
try {
PdfWriter.getInstance(document, new FileOutputStream(filePath));
document.open();
PdfPTable table = new PdfPTable(header.length);
table.setWidthPercentage(100);
for (String column : header) {
PdfPCell cell = new PdfPCell();
cell.setPhrase(new com.itextpdf.text.Paragraph(column));
table.addCell(cell);
}
for (int row = 0; row < data.length; row++) {
for (int column = 0; column < data[row].length; column++) {
PdfPCell cell = new PdfPCell();
cell.setPhrase(new com.itextpdf.text.Paragraph(data[row][column]));
table.addCell(cell);
}
}
document.add(table);
} catch (DocumentException e) {
e.printStackTrace();
} catch (FileNotFoundException e) {
e.printStackTrace();
} finally {
document.close();
}
}
public static void mergeCells(PdfPTable table, int row1, int col1, int row2, int col2) {
for (int row = row1; row <= row2; row++) {
for (int col = col1; col <= col2; col++) {
if (row == row1 && col == col1) {
continue;
}
PdfPCell cell = table.getRow(row).getCells()[col];
cell.setPhrase(null);
cell.setPadding(0);
cell.setBorder(PdfPCell.NO_BORDER);
}
}
PdfPCell cell = table.getRow(row1).getCells()[col1];
cell.setRowspan(row2 - row1 + 1);
cell.setColspan(col2 - col1 + 1);
}
}
```
调用 `exportTableToPDF` 方法可以将一个二维字符串数组导出为一个 PDF 表格文件,其中第一个参数是文件路径,第二个参数是表头数组,第三个参数是数据数组。
调用 `mergeCells` 方法可以将表格中的多个单元格合并为一个单元格,其中第一个参数是目标表格,第二个参数是起始行号,第三个参数是起始列号,第四个参数是结束行号,第五个参数是结束列号。
例如,如果要将第 2 行第 3、4、5 列合并为一个单元格,可以这样调用 `mergeCells` 方法:
```java
PDFExporter.mergeCells(table, 1, 2, 1, 4);
```
其中 `table` 是导出 PDF 表格的 `PdfPTable` 对象。
阅读全文
相关推荐
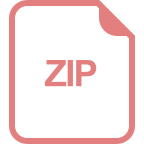
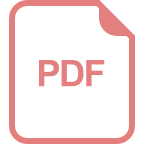
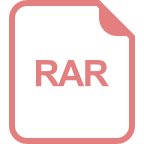
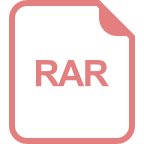
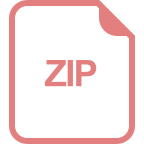
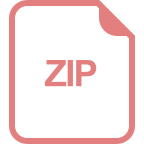
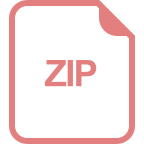
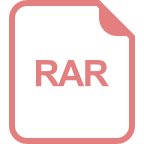
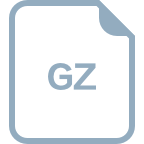
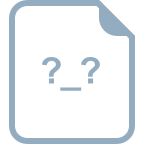
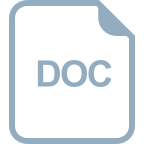

