iic eeprom verilog
时间: 2023-07-26 18:03:46 浏览: 121
您好!您的问题是关于 iic eeprom 的 Verilog 实现,对吗?那么,以下是一个简单的 iic eeprom 的 Verilog 代码示例,供您参考:
```verilog
module iic_eeprom(
input wire clk,
input wire rst,
input wire sda,
input wire scl,
input wire [7:0] addr,
input wire [7:0] data_in,
output wire [7:0] data_out,
output wire ack,
output wire done
);
reg [7:0] mem [0:255];
reg [7:0] rdata;
reg [7:0] wdata;
reg [7:0] cnt;
reg [7:0] addr_reg;
reg [2:0] state;
reg sda_reg;
reg scl_reg;
wire ack_reg;
/* IIC state machine */
parameter IDLE = 0, START = 1, ADDR = 2, DATA_IN = 3, DATA_OUT = 4, STOP = 5;
always @(posedge clk) begin
if (rst) begin
state <= IDLE;
cnt <= 0;
sda_reg <= 1;
scl_reg <= 1;
end else begin
case (state)
IDLE:
if (!scl && sda) begin
state <= START;
end
START:
sda_reg <= 0;
scl_reg <= 0;
if (cnt == 7) begin
state <= ADDR;
cnt <= 0;
addr_reg <= addr;
end else begin
cnt <= cnt + 1;
end
ADDR:
sda_reg <= addr_reg[cnt];
if (cnt == 7) begin
state <= DATA_IN;
cnt <= 0;
wdata <= data_in;
end else begin
cnt <= cnt + 1;
end
DATA_IN:
sda_reg <= wdata[cnt];
if (cnt == 7) begin
state <= ADDR;
cnt <= 0;
addr_reg <= addr;
ack <= 1;
end else begin
cnt <= cnt + 1;
end
DATA_OUT:
sda_reg <= rdata[cnt];
if (cnt == 7) begin
state <= STOP;
cnt <= 0;
end else begin
cnt <= cnt + 1;
end
STOP:
sda_reg <= 1;
scl_reg <= 1;
state <= IDLE;
endcase
end
end
/* IIC controller */
iic_controller iic_controller_inst(
.clk(clk),
.sda(sda),
.scl(scl),
.sda_reg(sda_reg),
.scl_reg(scl_reg),
.ack_reg(ack_reg)
);
/* IIC controller to read/write eeprom */
assign done = (state == STOP);
always @(posedge clk) begin
if (ack_reg) begin
case (state)
ADDR:
state <= DATA_OUT;
cnt <= 0;
DATA_IN:
state <= STOP;
cnt <= 0;
DATA_OUT:
rdata <= sda;
if (cnt == 7) begin
state <= START;
cnt <= 0;
end else begin
cnt <= cnt + 1;
end
endcase
end
end
/* eeprom memory */
always @(posedge clk) begin
if (state == DATA_IN) begin
mem[addr_reg] <= wdata;
end
if (state == DATA_OUT) begin
data_out <= mem[addr_reg];
end
end
endmodule
/* IIC controller */
module iic_controller(
input wire clk,
input wire sda,
input wire scl,
input wire sda_reg,
input wire scl_reg,
output wire ack_reg
);
reg sda_int;
reg scl_int;
always @(posedge clk) begin
sda_int <= sda_reg;
scl_int <= scl_reg;
end
assign ack_reg = (!sda && !scl);
assign sda = sda_int;
assign scl = scl_int;
endmodule
```
这个代码示例实现了一个简单的 iic eeprom,包括 iic 态机、iic 控制器、eeprom 存储器等功能。您可以根据自己的需求进行修改和优化。希望对您有所帮助!
阅读全文
相关推荐







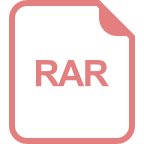









