请写一个关于xgboost机器学习模型调参的python代码
时间: 2023-08-31 17:31:39 浏览: 144
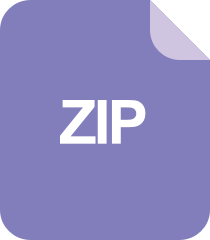
基于Python常用机器学习算法的简洁实现之XGboost.zip
### 回答1:
import xgboost as xgb
from sklearn.grid_search import GridSearchCV# 设置参数列表
param_grid = {
'max_depth': [3, 4, 5],
'learning_rate': [0.01, 0.1, 0.2],
'n_estimators': [200, 400, 600],
'subsample': [0.8, 1.0],
'colsample_bytree': [0.8, 1.0]
}
# 使用GridSearchCV进行搜索
xgb_model = xgb.XGBClassifier()
grid_search = GridSearchCV(xgb_model, param_grid, verbose=1, cv=5)
grid_search.fit(X_train, y_train)
# 输出最优参数
best_parameters = grid_search.best_params_
print(best_parameters)
### 回答2:
XGBoost是一种常用的梯度提升树算法,可以用于分类和回归问题。调参是优化模型性能的关键步骤。下面是一个关于XGBoost机器学习模型调参的Python代码示例:
```python
import xgboost as xgb
from sklearn.datasets import load_boston
from sklearn.model_selection import GridSearchCV, train_test_split
from sklearn.metrics import mean_squared_error
# 载入数据集
data = load_boston()
X, y = data.data, data.target
# 划分训练集和验证集
X_train, X_valid, y_train, y_valid = train_test_split(X, y, test_size=0.2, random_state=42)
# 定义模型
model = xgb.XGBRegressor()
# 定义要搜索的超参数范围
param_grid = {
'n_estimators': [50, 100, 200],
'max_depth': [3, 4, 5],
'learning_rate': [0.1, 0.01, 0.001]
}
# 网格搜索调参
grid = GridSearchCV(model, param_grid, scoring='neg_mean_squared_error', cv=5)
grid.fit(X_train, y_train)
# 输出最佳参数和最佳得分
print("Best Parameters: ", grid.best_params_)
print("Best Score: ", -grid.best_score_)
# 使用最佳参数的模型进行预测
best_model = grid.best_estimator_
y_pred = best_model.predict(X_valid)
# 计算均方误差
mse = mean_squared_error(y_valid, y_pred)
print("Mean Squared Error: ", mse)
```
在这个示例中,我们首先导入了必要的库,包括xgboost、sklearn.datasets等。然后我们使用`load_boston`函数载入一个波士顿房价的数据集,并将其划分为训练集和验证集。
接下来,我们定义了一个XGBoost回归模型,并定义了我们要搜索的超参数范围。在这个示例中,我们搜索了三个超参数:n_estimators(弱学习器的个数)、max_depth(树的最大深度)和learning_rate(学习率)。
然后,我们使用`GridSearchCV`函数进行网格搜索调参。其中,`scoring`参数指定了评估指标(负均方误差),`cv`参数指定了交叉验证的折数。
最后,我们输出了最佳参数和最佳得分。然后,使用最佳参数的模型进行预测,并计算了均方误差。
这是一个简单的示例,实际调参可能需要更多的超参数和更复杂的搜索策略,但以上代码可以作为一个起点帮助你进行XGBoost模型的调参。
### 回答3:
xgboost是一种强大的机器学习模型,但在使用过程中需要调参来优化模型的性能。下面是一个关于xgboost机器学习模型调参的Python代码示例:
```python
import xgboost as xgb
from sklearn.datasets import load_boston
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
from sklearn.model_selection import GridSearchCV
# 载入数据
boston = load_boston()
X, y = boston.data, boston.target
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
# 构建xgb模型
xgbr = xgb.XGBRegressor()
# 设置需要调参的参数
parameters = {'nthread': [4],
'objective': ['reg:squarederror'],
'learning_rate': [0.1, 0.01],
'max_depth': [3, 5, 7],
'min_child_weight': [1, 3, 5],
'subsample': [0.6, 0.8],
'colsample_bytree': [0.6, 0.8],
'n_estimators': [100, 200]
}
# 使用GridSearchCV进行调参
grid_search = GridSearchCV(estimator=xgbr, param_grid=parameters, scoring='neg_mean_squared_error', cv=5, n_jobs=-1)
grid_search.fit(X_train, y_train)
# 输出最佳参数和最佳得分
best_parameters = grid_search.best_params_
best_score = grid_search.best_score_
print("Best parameters: ", best_parameters)
print("Best score: ", best_score)
# 使用最佳参数训练模型
xgbr_best = xgb.XGBRegressor(**best_parameters)
xgbr_best.fit(X_train, y_train)
# 预测并计算均方误差
y_pred = xgbr_best.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
print("Mean Squared Error: ", mse)
```
以上代码使用了xgboost模型对波士顿房价数据进行预测,通过GridSearchCV调参获取最佳参数,并使用最佳参数训练模型,最后输出了预测结果的均方误差。你可以根据自己的需要,根据实际情况修改代码中的参数范围和评估指标。
阅读全文
相关推荐
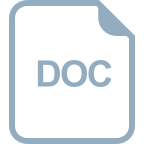
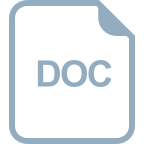
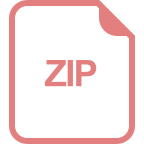



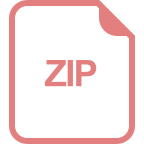
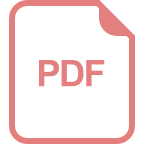
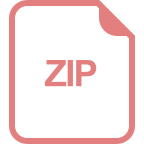
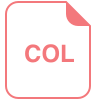
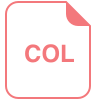






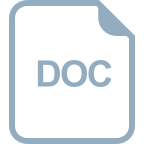