连接MySQL数据库, 可以进行增删改查
时间: 2023-11-03 21:15:01 浏览: 94
好的,连接 MySQL 数据库需要使用相应的驱动程序。以下是使用 Python 语言连接 MySQL 数据库并进行增删改查的示例代码:
首先,需要安装 MySQL 驱动程序,可以使用 pip 命令进行安装:
```
pip install mysql-connector-python
```
然后,先建立连接:
```python
import mysql.connector
# 建立连接
mydb = mysql.connector.connect(
host="localhost",
user="root",
password="password",
database="mydatabase"
)
# 获取游标
mycursor = mydb.cursor()
```
其中,`host` 为 MySQL 服务器地址,`user` 和 `password` 分别为用户名和密码,`database` 为要连接的数据库名。
接下来,可以进行增删改查操作,以下是示例代码:
```python
# 插入数据
sql = "INSERT INTO customers (name, address) VALUES (%s, %s)"
val = ("John", "Highway 21")
mycursor.execute(sql, val)
mydb.commit()
print(mycursor.rowcount, "record inserted.")
# 查询数据
mycursor.execute("SELECT * FROM customers")
myresult = mycursor.fetchall()
for x in myresult:
print(x)
# 更新数据
sql = "UPDATE customers SET address = %s WHERE address = %s"
val = ("Valley 345", "Highway 21")
mycursor.execute(sql, val)
mydb.commit()
print(mycursor.rowcount, "record(s) affected")
# 删除数据
sql = "DELETE FROM customers WHERE address = %s"
adr = ("Valley 345", )
mycursor.execute(sql, adr)
mydb.commit()
print(mycursor.rowcount, "record(s) deleted")
```
以上代码分别进行了插入数据、查询数据、更新数据和删除数据操作。执行完增删改操作后,需要调用 `mydb.commit()` 方法提交事务,否则数据不会被真正保存到数据库中。
注意:在实际开发中,需要根据具体业务需求编写 SQL 语句。同时,为了防止 SQL 注入攻击,应该使用参数化查询方式,而不是直接拼接 SQL 语句。
阅读全文
相关推荐
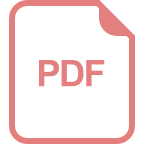
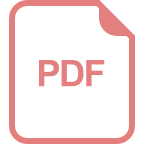
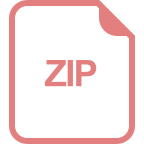
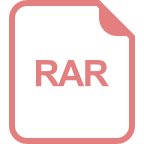
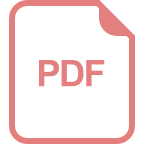
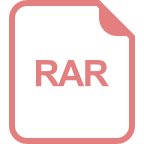
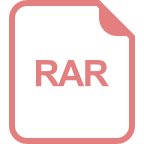
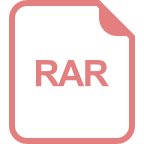
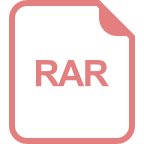
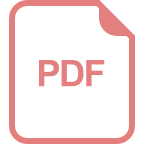
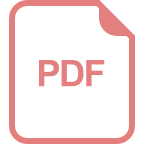
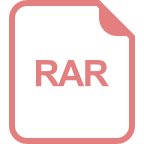
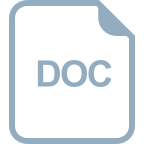
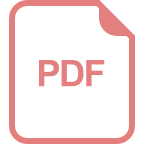