Python完成工程成本、工期与安全性多目标优化
时间: 2024-01-10 11:03:14 浏览: 78
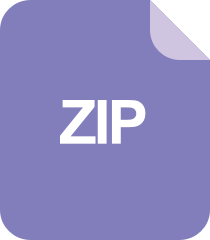
NSGAII算法的Python实现代码

Python可以使用多种优化算法来解决工程成本、工期与安全性多目标优化问题,例如遗传算法、粒子群算法、模拟退火算法等。以下是一个简单的使用遗传算法解决该问题的示例:
1. 定义目标函数
首先,需要定义一个目标函数,用于计算每个解的成本、工期和安全性指标。假设输入参数为一个长度为n的向量x,表示n个任务的完成时间,那么可以定义如下的目标函数:
```python
def objective(x):
cost = ... # 计算成本
duration = ... # 计算工期
safety = ... # 计算安全性指标
return cost, duration, safety
```
2. 定义遗传算法
接下来,需要定义一个遗传算法,用于优化目标函数。遗传算法是一种基于生物进化原理的优化算法,通过模拟自然选择、交叉和变异等过程来搜索最优解。以下是一个简单的遗传算法实现:
```python
import random
def genetic_algorithm(objective, n, pop_size, max_iter):
# 初始化种群
population = []
for i in range(pop_size):
x = [random.random() for j in range(n)]
population.append(x)
# 进化过程
for iter in range(max_iter):
# 计算适应度
fitness = [objective(x) for x in population]
# 选择操作
parents = []
for i in range(pop_size):
# 轮盘赌选择
idx1 = random.randint(0, pop_size-1)
idx2 = random.randint(0, pop_size-1)
parent1 = population[idx1]
parent2 = population[idx2]
if fitness[idx1] < fitness[idx2]:
parents.append(parent1)
else:
parents.append(parent2)
# 交叉操作
offspring = []
for i in range(pop_size):
idx1 = random.randint(0, pop_size-1)
idx2 = random.randint(0, pop_size-1)
parent1 = parents[idx1]
parent2 = parents[idx2]
child = []
for j in range(n):
if random.random() < 0.5:
child.append(parent1[j])
else:
child.append(parent2[j])
offspring.append(child)
# 变异操作
for i in range(pop_size):
for j in range(n):
if random.random() < 0.1:
offspring[i][j] = random.random()
# 更新种群
population = offspring
# 返回最优解
best_fitness = float('inf')
best_x = None
for x in population:
fitness = objective(x)
if fitness < best_fitness:
best_fitness = fitness
best_x = x
return best_x
```
3. 调用遗传算法
最后,可以使用定义好的目标函数和遗传算法来求解工程成本、工期和安全性多目标优化问题。例如,假设有10个任务需要完成,可以使用以下代码来调用遗传算法:
```python
n = 10
pop_size = 100
max_iter = 1000
best_x = genetic_algorithm(objective, n, pop_size, max_iter)
best_cost, best_duration, best_safety = objective(best_x)
print("Best solution: cost = %.2f, duration = %.2f, safety = %.2f" % (best_cost, best_duration, best_safety))
```
这样就可以得到一个近似最优的解,其中cost表示成本,duration表示工期,safety表示安全性指标。
阅读全文
相关推荐
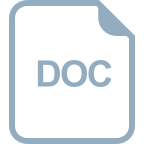
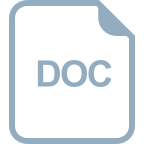
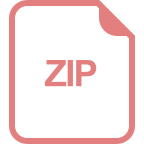
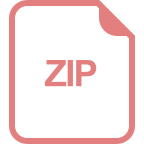
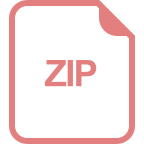
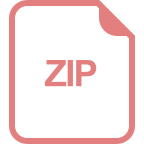
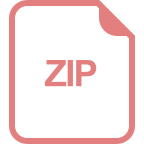
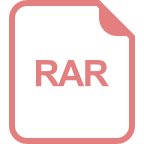
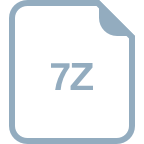
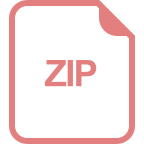
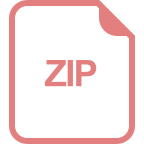
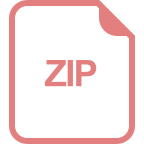
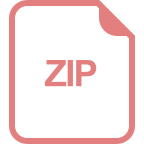
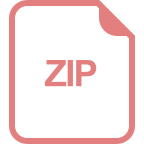
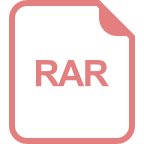
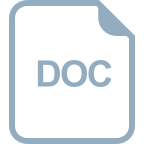
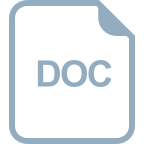