实现tableview的翻页功能,每页显示10行数据
时间: 2024-03-28 21:42:37 浏览: 63
您可以按照以下步骤来实现tableview的翻页功能,每页显示10行数据:
1. 首先,您需要确定要显示多少页数据。您可以通过计算数据源中的总行数并将其除以10来得到页数。
2. 然后,您需要跟踪当前显示的页数。您可以使用一个变量来存储当前页数,并在每次用户翻页时更新它。
3. 接下来,您需要在数据源方法中根据当前页数来返回对应的行数和单元格。
4. 在tableview的代理方法中,您可以使用当前页数来确定每个单元格的索引,并将其返回给tableview。
5. 最后,在用户翻页时,您只需要更新当前页数并重新加载tableview即可。
以下是一个示例代码,展示了如何实现tableview的翻页功能:
```swift
class ViewController: UIViewController, UITableViewDataSource, UITableViewDelegate {
@IBOutlet weak var tableView: UITableView!
var currentPage = 1
let pageSize = 10
var data = [Int]() // 假设数据源为一组整数
override func viewDidLoad() {
super.viewDidLoad()
// 初始化数据源
for i in 1...100 {
data.append(i)
}
// 设置tableview的代理和数据源
tableView.delegate = self
tableView.dataSource = self
tableView.rowHeight = 44
tableView.separatorStyle = .singleLine
}
// 返回总共有多少页数据
func numberOfPages() -> Int {
return Int(ceil(Double(data.count) / Double(pageSize)))
}
// 返回当前页应该显示的数据
func currentPageData() -> [Int] {
let start = (currentPage - 1) * pageSize
let end = min(start + pageSize, data.count)
return Array(data[start..<end])
}
// 数据源方法,返回当前页应该显示的行数
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return currentPageData().count
}
// 数据源方法,返回当前页应该显示的单元格
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath)
cell.textLabel?.text = "\(currentPageData()[indexPath.row])"
return cell
}
// tableview代理方法,返回当前页应该显示的单元格索引
func tableView(_ tableView: UITableView, willDisplay cell: UITableViewCell, forRowAt indexPath: IndexPath) {
let index = (currentPage - 1) * pageSize + indexPath.row
print("willDisplay cell at index \(index)")
}
// 用户翻页时更新当前页数并重新加载tableview
func nextPage() {
currentPage += 1
if currentPage > numberOfPages() {
currentPage = numberOfPages()
}
tableView.reloadData()
}
func prevPage() {
currentPage -= 1
if currentPage < 1 {
currentPage = 1
}
tableView.reloadData()
}
// 假设有两个按钮用于翻页
@IBAction func prevButtonPressed(_ sender: Any) {
prevPage()
}
@IBAction func nextButtonPressed(_ sender: Any) {
nextPage()
}
}
```
相关推荐
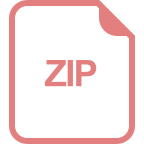








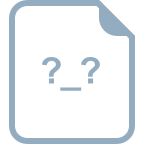
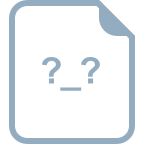
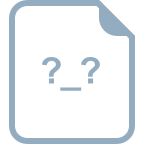
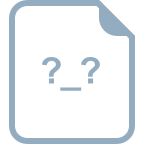
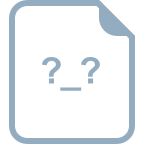