MATLAB代码实现CS直线裁剪算法,随机生成多条直线并用4*4大小的窗口进行裁剪
时间: 2024-03-21 08:38:25 浏览: 95
以下是MATLAB代码实现CS直线裁剪算法,随机生成多条直线并用4*4大小的窗口进行裁剪的示例:
```matlab
% 随机生成多条直线
num_lines = 10; % 直线数量
x = linspace(0, 1, 100); % x坐标
y = linspace(0, 1, 100); % y坐标
[X, Y] = meshgrid(x, y);
lines = zeros(num_lines, 4); % 存储每条直线的端点坐标
for i = 1:num_lines
x1 = rand();
y1 = rand();
x2 = rand();
y2 = rand();
lines(i, :) = [x1, y1, x2, y2];
end
% 绘制多条直线
figure;
hold on;
for i = 1:num_lines
plot([lines(i, 1), lines(i, 3)], [lines(i, 2), lines(i, 4)], '-');
end
axis([0, 1, 0, 1]);
title('原始图像');
% 用4*4大小的窗口进行裁剪
window_size = 4;
for i = 1:window_size:length(x)-window_size+1
for j = 1:window_size:length(y)-window_size+1
% 计算窗口范围
x1 = x(i);
y1 = y(j);
x2 = x(i+window_size-1);
y2 = y(j+window_size-1);
% 遍历每条直线,判断是否与窗口相交
for k = 1:num_lines
[is_intersect, x_intersect, y_intersect] = line_intersection(lines(k, 1), lines(k, 2), lines(k, 3), lines(k, 4), x1, y1, x2, y1);
if is_intersect
% 绘制被裁剪的直线段
plot([lines(k, 1), x_intersect], [lines(k, 2), y_intersect], 'r-');
end
[is_intersect, x_intersect, y_intersect] = line_intersection(lines(k, 1), lines(k, 2), lines(k, 3), lines(k, 4), x2, y1, x2, y2);
if is_intersect
% 绘制被裁剪的直线段
plot([lines(k, 1), x_intersect], [lines(k, 2), y_intersect], 'r-');
end
[is_intersect, x_intersect, y_intersect] = line_intersection(lines(k, 1), lines(k, 2), lines(k, 3), lines(k, 4), x1, y2, x2, y2);
if is_intersect
% 绘制被裁剪的直线段
plot([lines(k, 1), x_intersect], [lines(k, 2), y_intersect], 'r-');
end
[is_intersect, x_intersect, y_intersect] = line_intersection(lines(k, 1), lines(k, 2), lines(k, 3), lines(k, 4), x1, y1, x1, y2);
if is_intersect
% 绘制被裁剪的直线段
plot([lines(k, 1), x_intersect], [lines(k, 2), y_intersect], 'r-');
end
end
end
end
% 判断直线是否与矩形相交
function [is_intersect, x_intersect, y_intersect] = line_intersection(x1, y1, x2, y2, x3, y3, x4, y4)
is_intersect = false;
x_intersect = 0;
y_intersect = 0;
% 计算直线1和直线2的斜率和截距
if x1 == x2
k1 = Inf;
b1 = x1;
else
k1 = (y2 - y1) / (x2 - x1);
b1 = y1 - k1 * x1;
end
if x3 == x4
k2 = Inf;
b2 = x3;
else
k2 = (y4 - y3) / (x4 - x3);
b2 = y3 - k2 * x3;
end
% 判断是否相交
if k1 == k2
return;
end
if isinf(k1) || isinf(k2)
if isinf(k1)
x_intersect = b1;
y_intersect = k2 * x_intersect + b2;
else
x_intersect = b2;
y_intersect = k1 * x_intersect + b1;
end
else
x_intersect = (b2 - b1) / (k1 - k2);
y_intersect = k1 * x_intersect + b1;
end
if x_intersect >= min(x1, x2) && x_intersect <= max(x1, x2) && ...
x_intersect >= min(x3, x4) && x_intersect <= max(x3, x4) && ...
y_intersect >= min(y1, y2) && y_intersect <= max(y1, y2) && ...
y_intersect >= min(y3, y4) && y_intersect <= max(y3, y4)
is_intersect = true;
end
end
```
其中,`line_intersection`函数用于判断直线是否与矩形相交,`is_intersect`表示是否相交,`x_intersect`和`y_intersect`表示交点的坐标。在使用窗口进行裁剪时,我们遍历每个窗口,并判断每条直线是否与窗口相交,如果相交则将相交的部分绘制出来。
阅读全文
相关推荐

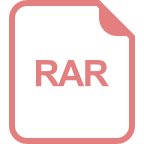













