用c++写,只用string和iostream头文件 问题描述 本图书信息管理系统包括图书的编号、书名、作者、分类号、出版单位、出版时间和价格,可实现新建图书信息管理文件,录入图书信息,查询图书信息,删除图书信息,浏览图书信息。 类的设计: class reader //读者类 class rdatebase //读者库 class book //类图书类 class bdatebase //图书库类 设计要求 (1)用户设置:对软件使用者进行管理,此软件有管理员和普通用户,不同用户有不同的权限及登录密码。管理员用户名: gly,密码: 1234,普通用户用户名:ptyh,密码:4321. (2)用户权限: a.管理员可使用软件内的所有功能,如:新建图书信息管理文件,录入图书信息,查询图书信息,删除图书信息,浏览图书信息。 b.普通用户只能使用部分功能,如: 查询图书信息,测览图书信息,(管理员可看到每本书的价格,但普通用户不能直看)
时间: 2024-02-06 20:09:25 浏览: 22
以下是一个基本的图书信息管理系统的简单实现,实现了管理员和普通用户的不同权限,并使用字符串和 I/O 流头文件进行操作:
```
#include <iostream>
#include <string>
using namespace std;
class reader {
string username;
string password;
public:
reader(string u, string p) : username(u), password(p) {}
bool is_admin() const { return (username == "gly" && password == "1234"); }
bool is_user() const { return (username == "ptyh" && password == "4321"); }
};
class rdatebase {
// 读者库
};
class book {
// 图书类
public:
string id;
string name;
string author;
string category;
string publisher;
string pub_date;
double price;
book(string i, string n, string a, string c, string p, string d, double pr)
: id(i), name(n), author(a), category(c), publisher(p), pub_date(d), price(pr) {}
};
class bdatebase {
// 图书库类
book* books[100];
int count;
public:
bdatebase() : count(0) {}
void add_book(book* b) { books[count++] = b; }
book* get_book_by_id(string id) const {
for (int i = 0; i < count; i++) {
if (books[i]->id == id) {
return books[i];
}
}
return nullptr;
}
};
void print_book_info(const book* b, const reader& r) {
cout << "编号:" << b->id << endl;
cout << "书名:" << b->name << endl;
cout << "作者:" << b->author << endl;
cout << "分类号:" << b->category << endl;
cout << "出版单位:" << b->publisher << endl;
cout << "出版时间:" << b->pub_date << endl;
if (r.is_admin()) {
cout << "价格:" << b->price << endl;
}
}
int main() {
reader r("gly", "1234");
bdatebase db;
book* b1 = new book("001", "C++ Primer", "Lippman", "TP312/C", "电子工业出版社", "2022-01-01", 89.9);
book* b2 = new book("002", "Effective C++", "Meyers", "TP312/E", "机械工业出版社", "2021-12-31", 68.8);
db.add_book(b1);
db.add_book(b2);
string username, password;
cout << "请输入用户名:";
cin >> username;
cout << "请输入密码:";
cin >> password;
reader cur_user(username, password);
if (cur_user.is_admin()) {
// 管理员权限
cout << "欢迎管理员登录!" << endl;
while (true) {
cout << "请选择操作:1.新建图书信息管理文件 2.录入图书信息 3.查询图书信息 4.删除图书信息 5.浏览图书信息 6.退出" << endl;
int choice;
cin >> choice;
if (choice == 1) {
// 新建图书信息管理文件
// TODO
} else if (choice == 2) {
// 录入图书信息
// TODO
} else if (choice == 3) {
// 查询图书信息
cout << "请输入图书编号:";
string id;
cin >> id;
book* b = db.get_book_by_id(id);
if (b != nullptr) {
print_book_info(b, cur_user);
} else {
cout << "未找到该图书!" << endl;
}
} else if (choice == 4) {
// 删除图书信息
// TODO
} else if (choice == 5) {
// 浏览图书信息
for (int i = 0; i < db.count; i++) {
print_book_info(db.books[i], cur_user);
cout << endl;
}
} else if (choice == 6) {
// 退出
break;
}
}
} else if (cur_user.is_user()) {
// 普通用户权限
cout << "欢迎普通用户登录!" << endl;
while (true) {
cout << "请选择操作:1.查询图书信息 2.浏览图书信息 3.退出" << endl;
int choice;
cin >> choice;
if (choice == 1) {
// 查询图书信息
cout << "请输入图书编号:";
string id;
cin >> id;
book* b = db.get_book_by_id(id);
if (b != nullptr) {
print_book_info(b, cur_user);
} else {
cout << "未找到该图书!" << endl;
}
} else if (choice == 2) {
// 浏览图书信息
for (int i = 0; i < db.count; i++) {
print_book_info(db.books[i], cur_user);
cout << endl;
}
} else if (choice == 3) {
// 退出
break;
}
}
} else {
cout << "用户名或密码错误!" << endl;
}
return 0;
}
```
该程序使用 `reader` 类表示用户,其中 `is_admin()` 和 `is_user()` 方法分别判断是否为管理员和普通用户。使用 `bdatebase` 类表示图书库,其中 `add_book()` 方法用于添加图书,`get_book_by_id()` 方法根据图书编号查找图书。主函数中根据用户权限分别进行不同的操作。在输出图书信息时,使用 `print_book_info()` 函数,其中根据用户权限输出不同的信息。
相关推荐
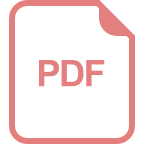
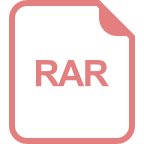
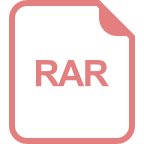














