如何设置QComboBox点击某项后下拉列表不消失
时间: 2024-02-25 22:53:18 浏览: 31
要设置QComboBox点击某项后下拉列表不消失,可以使用QComboBox的view()方法获取其下拉列表的视图对象,然后设置其属性,如下所示:
```
comboBox.view().setMouseTracking(True)
comboBox.view().setAutoFillBackground(False)
comboBox.view().setStyleSheet("QListView::item:hover{background-color:#F0F8FF;}")
```
其中,setMouseTracking(True)用于开启鼠标追踪,setAutoFillBackground(False)用于关闭自动填充背景色,setStyleSheet()用于设置鼠标悬停时的背景颜色。这样设置后,下拉列表就不会在点击某项后立即消失了。
相关问题
如何设置QComboBox下拉列表不消失
要设置QComboBox下拉列表不消失,可以使用QComboBox的setView()方法设置一个自定义的QListView视图,然后在该视图对象中重写mouseReleaseEvent()方法,使其不会在鼠标释放时关闭下拉列表。
具体的实现代码如下:
```python
from PyQt5.QtWidgets import QApplication, QComboBox, QListView
class MyComboBox(QComboBox):
def __init__(self, parent=None):
super(MyComboBox, self).__init__(parent)
self.setView(QListView(self))
def view(self):
return super(MyComboBox, self).view()
def mouseReleaseEvent(self, event):
index = self.view().currentIndex()
if index.isValid():
self.setCurrentIndex(index.row())
self.view().hide()
if __name__ == '__main__':
app = QApplication([])
combo = MyComboBox()
combo.addItems(['item1', 'item2', 'item3'])
combo.show()
app.exec_()
```
在这个例子中,我们自定义了一个MyComboBox类,它继承自QComboBox类,并重写了mouseReleaseEvent()方法。在该方法中,我们首先获取当前选中的项的索引,然后将其设为当前项,并隐藏下拉列表。
通过这种方式,我们就可以实现QComboBox下拉列表不消失的效果。
如何用qt实现QComboBox下拉列表不消失
要用Qt实现QComboBox下拉列表不消失,可以使用QComboBox的view()方法获取其下拉列表的视图对象,然后在该视图对象中重写mouseReleaseEvent()方法,使其不会在鼠标释放时关闭下拉列表。
具体的实现代码如下:
```cpp
#include <QApplication>
#include <QComboBox>
#include <QListView>
#include <QDebug>
class MyComboBox : public QComboBox
{
public:
MyComboBox(QWidget *parent = nullptr) : QComboBox(parent)
{
setView(new QListView(this));
}
QListView* view() const
{
return qobject_cast<QListView*>(QComboBox::view());
}
protected:
void mouseReleaseEvent(QMouseEvent *event) override
{
int index = view()->currentIndex().row();
if (index >= 0) {
setCurrentIndex(index);
}
view()->hide();
}
};
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MyComboBox comboBox;
comboBox.addItems({"item1", "item2", "item3"});
comboBox.show();
return a.exec();
}
```
在这个例子中,我们自定义了一个MyComboBox类,它继承自QComboBox类,并重写了mouseReleaseEvent()方法。在该方法中,我们首先获取当前选中的项的索引,然后将其设为当前项,并隐藏下拉列表。
通过这种方式,我们就可以用Qt实现QComboBox下拉列表不消失的效果。
相关推荐
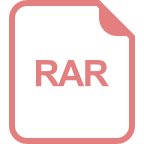












