python图片字符分割白底黑字
时间: 2023-07-05 10:29:39 浏览: 246
您好!针对您的问题,可以使用以下步骤进行图片字符分割:
1. 将图片进行灰度化和二值化处理,使得图片的背景为白色,字符为黑色。
```python
import cv2
img = cv2.imread('image.png')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)[1]
```
2. 对二值化后的图片进行轮廓检测,得到每个字符的轮廓。
```python
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
```
3. 对每个字符的轮廓进行排序和裁剪,得到每个字符的图像。
```python
contours = sorted(contours, key=lambda c: cv2.boundingRect(c)[0])
for contour in contours:
(x, y, w, h) = cv2.boundingRect(contour)
roi = img[y:y + h, x:x + w]
```
4. 保存每个字符的图像。
```python
cv2.imwrite('character.png', roi)
```
希望这能帮到您!
相关问题
python对已经灰度化过的白底黑字图片进行字符分割
好的,针对您的问题,可以使用以下步骤对已经灰度化的白底黑字图片进行字符分割:
1. 首先,对已经灰度化的图片进行二值化处理,使得图片的背景为白色,字符为黑色。
```python
import cv2
img = cv2.imread('image.png', 0)
ret, thresh = cv2.threshold(img, 127, 255, cv2.THRESH_BINARY_INV)
```
2. 对二值化后的图片进行轮廓检测,得到每个字符的轮廓。
```python
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
```
3. 对每个字符的轮廓进行排序和裁剪,得到每个字符的图像。
```python
contours = sorted(contours, key=lambda c: cv2.boundingRect(c)[0])
for contour in contours:
(x, y, w, h) = cv2.boundingRect(contour)
roi = img[y:y + h, x:x + w]
```
4. 保存每个字符的图像。
```python
cv2.imwrite('character.png', roi)
```
希望这能帮到您!
在图像处理中,用python语言设计一个算法,对任意输入的数字的图像(规定输入只能是0-9之中的一个,且为白底黑字的图片)进行识别,得出该数字的识别结果
在Python中设计一个简单的数字图像识别算法,我们可以使用OpenCV库进行图像预处理,然后结合像Tesseract OCR(Optical Character Recognition,光学字符识别)这样的OCR工具来进行字符识别。以下是一个基本步骤的概述:
1. **导入库**:
首先,安装必要的库,如`opencv-python`, `numpy`, 和 `pytesseract` (通过pip install命令)。
```python
import cv2
import numpy as np
from PIL import Image
import pytesseract
```
2. **读取和预处理图像**:
使用OpenCV读取图像,并进行灰度化、二值化等操作以便于后续处理。
```python
def preprocess_image(image_path):
img = cv2.imread(image_path, 0)
_, thresholded = cv2.threshold(img, 127, 255, cv2.THRESH_BINARY_INV) # 可能需要调整阈值
return thresholded
```
3. **分割字符**:
对图像进行切割,通常每个数字是一个独立的对象,可以使用轮廓检测或模板匹配找出单独的字符区域。
```python
def segment_chars(thresholded_img):
contours, _ = cv2.findContours(thresholded_img, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
chars = []
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
char = thresholded_img[y:y+h, x:x+w]
chars.append(char)
return chars
```
4. **应用OCR**:
将分割出的字符送入Tesseract进行识别,获取文本内容。
```python
def recognize_char(chars, lang="eng"):
text = ""
for char in chars:
pil_image = Image.fromarray(char)
result = pytesseract.image_to_string(pil_image, lang=lang)
if result.strip():
text += result
return text
```
5. **主函数整合**:
调用上述函数并处理整个流程。
```python
def main(image_path):
processed_img = preprocess_image(image_path)
segmented_chars = segment_chars(processed_img)
recognized_text = recognize_char(segmented_chars)
return recognized_text
# 使用示例
image_path = 'path/to/your/image.png'
result = main(image_path)
print(f"识别结果: {result}")
```
**相关问题--**:
1. 识别率如何提升?
2. 这种方法是否适用于彩色图像?
3. 如何处理手写字体的识别?
阅读全文
相关推荐
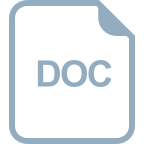
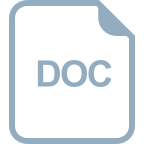
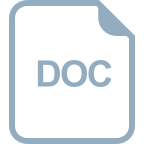
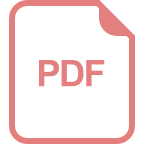
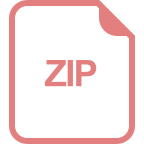
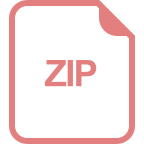

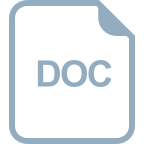
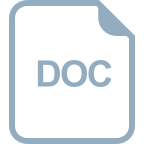
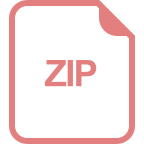
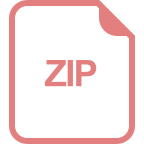
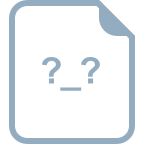
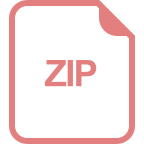
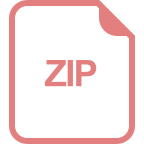
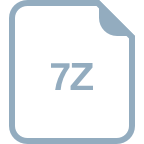
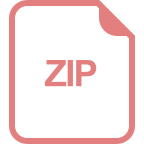