怎么调用String str = "Hello world, this is a Java program."; countWords(str);
时间: 2024-03-16 12:41:09 浏览: 14
您可以使用以下代码来调用 `countWords` 方法:
```java
String str = "Hello world, this is a Java program.";
int count = countWords(str);
System.out.println("The number of words in the string is: " + count);
```
其中 `countWords` 方法的实现可能如下所示:
```java
public static int countWords(String str) {
if (str == null || str.isEmpty()) {
return 0;
}
String[] words = str.split("\\s+");
return words.length;
}
```
该方法将字符串参数按空格分割成单词数组,然后返回单词数组的长度(即单词数)作为结果。在上述示例中,将字符串 `"Hello world, this is a Java program."` 传递给 `countWords` 方法,然后将返回值存储在 `count` 变量中,并将其输出到控制台。
相关问题
键盘输入任意的三个字符串,实现文本的查找替换功能。如: String source="This is Java program, Java is good ......."; String find="Java"; String dest="Java language"; 要求: (1)将source串中的第一个字符串 find,用字符串dest替换。 (2)仅替换最后的一个串find (3)将source中的所有 find串替换为字符串 dest。 (4)统计 source串中共有多少个单词。
下面是Java代码实现:
```
import java.util.Scanner;
public class TextManipulation {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入第一个字符串:");
String source = scanner.nextLine();
System.out.print("请输入第二个字符串:");
String find = scanner.nextLine();
System.out.print("请输入第三个字符串:");
String dest = scanner.nextLine();
// (1)将source串中的第一个字符串find,用字符串dest替换。
String result1 = source.replaceFirst(find, dest);
System.out.println("替换第一个字符串:" + result1);
// (2)仅替换最后的一个串find
String result2 = source.substring(0, source.lastIndexOf(find)) + dest + source.substring(source.lastIndexOf(find) + find.length());
System.out.println("替换最后的一个字符串:" + result2);
// (3)将source中的所有find串替换为字符串dest。
String result3 = source.replaceAll(find, dest);
System.out.println("替换所有字符串:" + result3);
// (4)统计source串中共有多少个单词
int count = countWords(source);
System.out.println("共有" + count + "个单词");
}
public static int countWords(String str) {
if (str == null || str.isEmpty()) {
return 0;
}
char[] chars = str.toCharArray();
int count = 0;
boolean isWord = false;
for (int i = 0; i < chars.length; i++) {
if (chars[i] != ' ') {
if (!isWord) {
isWord = true;
count++;
}
} else {
isWord = false;
}
}
return count;
}
}
```
首先从键盘输入三个字符串 source、find、dest,然后按照题目要求依次进行字符串的查找替换和单词统计。
其中第一个字符串 find 只替换第一个出现的位置,可以使用 String 类的 replaceFirst 方法;最后一个字符串 find 可以使用 String 类的 substring 方法和 lastIndexOf 方法进行查找和替换;所有字符串 find 可以使用 String 类的 replaceAll 方法进行替换;单词统计可以使用之前提到的方法实现。
import java.util.Scanner; import java.util.StringTokenizer; public class CountWords { public static void main(String[] args) { //输入文章内容(设文章内容为 一行字符) Scanner sc = new Scanner(System.in); String text = sc.nextLine(); //设置分隔符的正则表达式 【】 //方法1:用正则表达式实现字符串拆分 【】 System.out.println("\n单词数:"+【】); //方法2:用StringTokenizer实现字符串解析 【】 System.out.println("\n单词数:"+【】); } }
您好,这段代码是一个统计单词数的Java程序,需要您填充其中的部分代码以实现功能。以下是完整的代码示例,其中已经填充了缺失的部分:
```java
import java.util.Scanner;
import java.util.StringTokenizer;
public class CountWords {
public static void main(String[] args) {
//输入文章内容(设文章内容为 一行字符)
Scanner sc = new Scanner(System.in);
String text = sc.nextLine();
//设置分隔符的正则表达式
String regex = "\\s+|\\pP";
//方法1:用正则表达式实现字符串拆分
String[] words = text.split(regex);
System.out.println("\n单词数:"+words.length);
//方法2:用StringTokenizer实现字符串解析
StringTokenizer st = new StringTokenizer(text, regex);
System.out.println("\n单词数:"+st.countTokens());
}
}
```
在这个程序中,首先使用Scanner类从标准输入读取文章内容,然后设置了一个包括空格和标点符号的正则表达式作为分隔符。接下来,使用了两种不同的方法来统计文章中的单词数。
第一个方法使用了String类的split()方法,通过传入正则表达式将文章按照分隔符进行拆分成一个单词数组,然后输出数组长度即为单词数。
第二个方法使用了StringTokenizer类,将文章和分隔符传入构造函数创建一个StringTokenizer对象,然后使用countTokens()方法统计单词数量。
希望这个程序能够帮助您理解如何统计英文单词数。
相关推荐
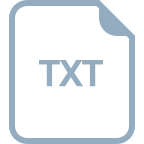
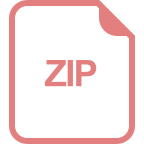
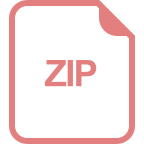












