1. Given: Three DNA strings in DNA.txt file Return: for each sequence 1) the complementary strand . 2) GC content. And store the output in the same file using attach model. (If you can’t write the loops, read the file or attach the file, just do it as far as you can.)
时间: 2024-03-10 22:46:55 浏览: 61
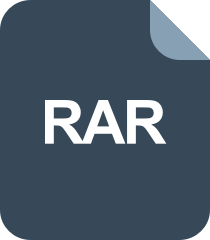
解决linux下出现/lib64/libstdc++.so.6: version `CXXABI_1.3.8' not found问题
假设`DNA.txt`中包含三个DNA序列,每个序列都以一行文本的形式存储。我们可以按照以下方式读取该文件并处理序列:
```python
# 打开文件
with open('DNA.txt', 'r') as file:
# 读取所有行
lines = file.readlines()
# 遍历每个序列
for i in range(3):
# 读取当前序列的字符串
dna = lines[i].strip()
# 计算GC含量
gc_content = (dna.count('G') + dna.count('C')) / len(dna) * 100
# 计算互补序列
complement = ''
for base in dna:
if base == 'A':
complement += 'T'
elif base == 'T':
complement += 'A'
elif base == 'C':
complement += 'G'
elif base == 'G':
complement += 'C'
# 输出结果到文件
with open('DNA.txt', 'a') as output_file:
output_file.write(f'Sequence {i+1}: Complement: {complement}, GC content: {gc_content:.2f}%\n')
```
上面的代码首先使用`open()`函数打开`DNA.txt`文件,并使用`readlines()`方法读取文件中的所有行。然后,我们使用一个循环遍历每个序列,并使用`strip()`方法去除每个序列字符串开头和结尾的空格。
接着,我们计算序列的GC含量。我们可以使用字符串的`count()`方法来计算G和C的数量,并将它们除以序列的长度,乘以100,即可计算出GC含量。
然后,我们计算序列的互补序列。我们可以使用一个循环遍历序列中的每个碱基,并根据碱基的类型,将其替换为互补碱基。
最后,我们将结果输出到`DNA.txt`文件中。我们使用`open()`函数打开文件,并将其模式设置为附加模式(`'a'`),这样我们可以将新的结果添加到文件的末尾。然后,我们使用`write()`方法将结果字符串写入文件中。
需要注意的是,如果我们直接将结果写入到`DNA.txt`文件中,则可能会覆盖该文件中原有的内容。为了避免这种情况,我们可以将结果写入到一个新的文件中,或者使用模式为附加模式的`open()`函数来打开文件并将结果附加到文件末尾。
阅读全文
相关推荐
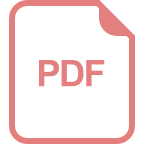
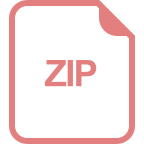















