//使用string类型输入一个引文语句,试编写一个函数提取出语句中的所有单词, //并在main函数中输出所有单词,要求每行输出一个单词
时间: 2023-09-26 11:06:43 浏览: 104
### 回答1:
好的,我可以回答这个问题。以下是提取单词的函数代码:
```
#include <iostream>
#include <string>
#include <sstream>
using namespace std;
void extractWords(string sentence) {
stringstream ss(sentence);
string word;
while (ss >> word) {
cout << word << endl;
}
}
int main() {
string sentence;
getline(cin, sentence);
extractWords(sentence);
return ;
}
```
这个函数会将输入的引文语句中的所有单词提取出来,并在 main 函数中每行输出一个单词。
### 回答2:
可以定义一个函数extractWords来提取句子中的所有单词,算法如下:
1. 定义一个空字符串变量word,用来保存当前提取的单词。
2. 遍历输入的句子中的每一个字符:
- 如果该字符是字母,则追加到word中;
- 如果该字符是空格或标点符号,则判断word是否为空:
- 如果word不为空,则输出word,然后将word重置为空字符串。
3. 如果句子的最后一个字符不是空格或标点符号,则需要在结束前输出word。
下面是这个函数的示例代码:
#include <iostream>
#include <string>
using namespace std;
void extractWords(string sentence) {
string word = "";
for (char c : sentence) {
if (isalpha(c)) {
word += c;
} else if (!word.empty()) {
cout << word << endl;
word = "";
}
}
if (!word.empty()) {
cout << word << endl;
}
}
int main() {
string sentence;
cout << "请输入一个引文语句:";
getline(cin, sentence);
cout << "提取的单词为:" << endl;
extractWords(sentence);
return 0;
}
在main函数中首先输入一个引文语句,然后调用extractWords函数提取出其中的所有单词,并逐行输出。
### 回答3:
下面是一个提取单词并按行输出的函数实现:
```python
def extract_words(sentence):
# 将句子按空格分割成单词列表
words = sentence.split(" ")
# 遍历单词列表,输出每个单词
for word in words:
print(word)
# 在主函数中调用提取单词的函数并传入引文语句
def main():
sentence = input("请输入一个引文语句:")
extract_words(sentence)
main()
```
这是一个简单的实现,按照空格分割句子为单词列表,然后遍历输出每个单词。每行输出一个单词。请注意,这个函数假设句子中的单词是由空格分隔的,如果句子中使用了其他符号(如标点符号等)来分隔单词,还需要进行相应的处理。
阅读全文
相关推荐
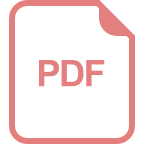
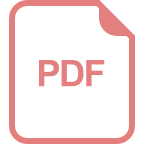
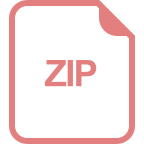
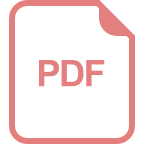
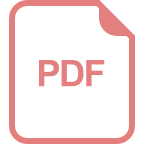
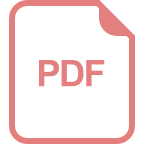
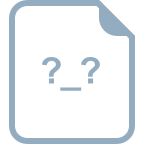
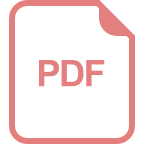
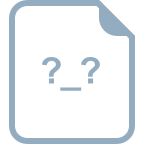
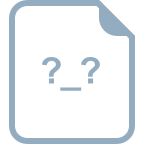
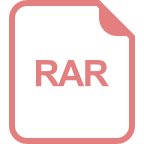
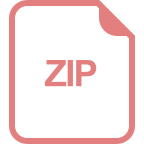
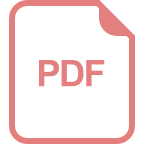
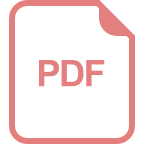
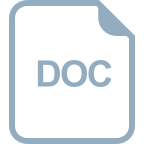
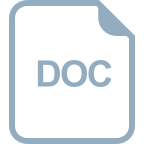