用matlab写一个冒泡程序
时间: 2023-02-25 18:19:22 浏览: 210
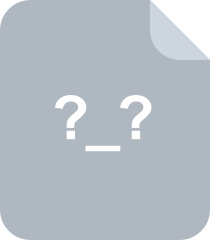
matlab冒泡排序
下面是一个使用 Matlab 编写的冒泡排序程序的代码示例:
```
function y = bubble_sort(x)
n = length(x);
for i = 1 : n-1
for j = 1 : n-i
if x(j) > x(j+1)
temp = x(j);
x(j) = x(j+1);
x(j+1) = temp;
end
end
end
y = x;
end
```
使用方法:输入 `x` 向量作为参数,运行此函数,结果返回为从小到大排序后的向量 `y`。
阅读全文
相关推荐

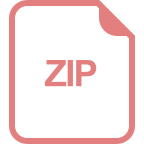

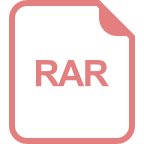
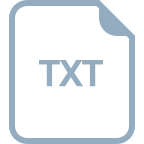
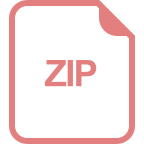
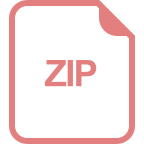
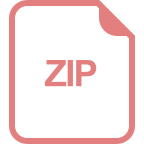
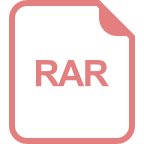
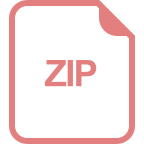
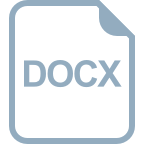
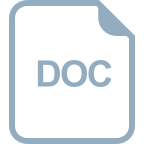
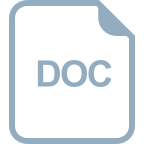