通过Servlet得到数据库中的数据并显示在浏览器端
时间: 2024-03-06 13:49:07 浏览: 18
要通过Servlet得到数据库中的数据并显示在浏览器端,可以按照以下步骤进行:
1. 在Servlet中引入需要的java.sql包,以便通过JDBC连接数据库。
2. 在Servlet中编写代码,连接数据库,执行SQL语句,将数据查询出来。
3. 将查询出来的数据存储在request对象中,以便在JSP页面中进行显示。
4. 在JSP页面中通过EL表达式或JSTL标签来获取Servlet中存储的数据,并进行页面展示。
以下是一个示例代码,可以参考:
Servlet代码:
```java
import java.sql.*;
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
public class DisplayDataServlet extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// JDBC连接数据库
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "root";
String password = "mypassword";
String driver = "com.mysql.jdbc.Driver";
Connection con = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
Class.forName(driver);
con = DriverManager.getConnection(url, user, password);
// 执行SQL语句,查询数据
String sql = "select * from mytable";
pstmt = con.prepareStatement(sql);
rs = pstmt.executeQuery();
// 将查询结果存储在request对象中
request.setAttribute("result", rs);
} catch (Exception e) {
e.printStackTrace();
} finally {
try { rs.close(); } catch (Exception e) { }
try { pstmt.close(); } catch (Exception e) { }
try { con.close(); } catch (Exception e) { }
}
// 转发到JSP页面进行显示
RequestDispatcher dispatcher = request.getRequestDispatcher("/displaydata.jsp");
dispatcher.forward(request, response);
}
}
```
JSP页面代码(displaydata.jsp):
```html
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Display Data</title>
</head>
<body>
<table>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
</tr>
<c:forEach var="row" items="${result}">
<tr>
<td><c:out value="${row.id}" /></td>
<td><c:out value="${row.name}" /></td>
<td><c:out value="${row.age}" /></td>
</tr>
</c:forEach>
</table>
</body>
</html>
```
在以上示例代码中,Servlet中连接了名为“mydatabase”的MySQL数据库,并查询了名为“mytable”的数据表中的所有记录。查询结果存储在request对象中,并通过转发到名为“displaydata.jsp”的JSP页面进行显示。在JSP页面中,使用了JSTL标签<c:forEach>来遍历查询结果,并通过<c:out>标签将数据显示在HTML表格中。
相关推荐
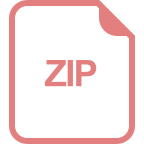
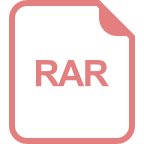
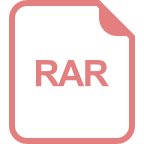















