编写一个Java应用程序,绘制转动的风扇
时间: 2024-06-09 10:06:31 浏览: 93
以下是一个简单的Java应用程序,可以绘制旋转的风扇,你可以根据自己的需要进行修改和调整。
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Fan extends JFrame {
private FanPanel fanPanel;
public static void main(String[] args) {
Fan fan = new Fan();
fan.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
fan.setSize(400, 400);
fan.setVisible(true);
}
public Fan() {
super("Fan");
fanPanel = new FanPanel();
add(fanPanel);
}
private class FanPanel extends JPanel implements ActionListener {
private Timer timer;
private int angle;
public FanPanel() {
timer = new Timer(10, this);
timer.start();
}
public void actionPerformed(ActionEvent e) {
angle += 1;
if (angle >= 360) {
angle = 0;
}
repaint();
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
int x = getWidth() / 2;
int y = getHeight() / 2;
int radius = Math.min(x, y) - 20;
int[] xPoints = new int[4];
int[] yPoints = new int[4];
for (int i = 0; i < 4; i++) {
xPoints[i] = x + (int)(radius * Math.cos(Math.toRadians(angle + i * 90)));
yPoints[i] = y - (int)(radius * Math.sin(Math.toRadians(angle + i * 90)));
}
g.setColor(Color.RED);
g.fillPolygon(xPoints, yPoints, 4);
g.setColor(Color.BLACK);
g.drawOval(x - radius, y - radius, radius * 2, radius * 2);
}
}
}
```
代码中使用了一个 `JPanel` 来绘制风扇,通过一个 `Timer` 定时器来定时更新风扇的角度。在 `paintComponent` 方法中,通过计算三角函数来确定风扇的四个点的坐标,并使用 `fillPolygon` 方法来绘制风扇的叶片。最后使用 `drawOval` 方法来绘制风扇的圆形轮廓。
阅读全文
相关推荐
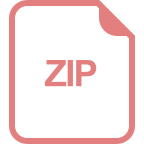
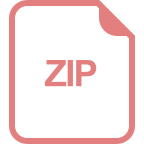
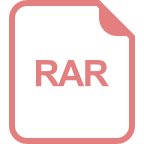
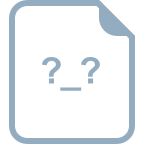
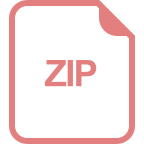
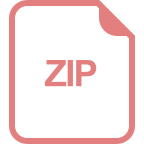
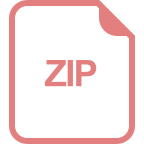
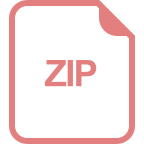
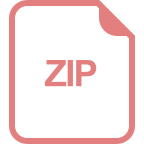
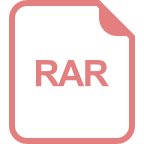
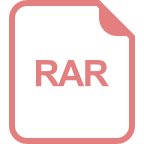
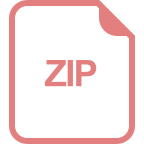
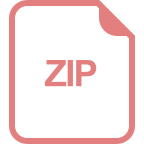
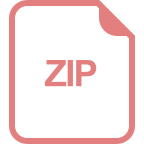