操作系统里生产者消费者问题c语言代码
时间: 2024-11-22 08:25:44 浏览: 80
操作系统中的生产者消费者问题是经典的并发控制问题,它模拟了一个生产者(Producer)负责生成数据并放入缓冲区,而消费者(Consumer)负责从缓冲区消费数据的情况。下面是一个简单的基于C语言的生产者消费者问题的线程同步实现,这里我们使用互斥锁(mutex)和条件变量(condition variable):
```c
#include <pthread.h>
#include <stdio.h>
#define BUFFER_SIZE 5
#define EMPTY 0
#define FULL 1
typedef struct {
int data[BUFFER_SIZE];
int count;
int state; // 状态标志,表示当前状态(空或满)
pthread_mutex_t lock; // 互斥锁
pthread_cond_t empty; // 当缓冲区为空时等待的信号
pthread_cond_t full; // 当缓冲区满时等待的信号
} Buffer;
void* producer(void* arg) {
Buffer* buffer = (Buffer*)arg;
int i;
while (1) {
pthread_mutex_lock(&buffer->lock);
while (buffer->count == BUFFER_SIZE) { // 如果缓冲区已满,等待
pthread_cond_wait(&buffer->full, &buffer->lock);
}
// 生产数据
buffer->data[buffer->count] = i++;
buffer->count++;
printf("Produced: %d\n", buffer->data[buffer->count - 1]);
if (buffer->count == 1) { // 设置状态变为非空
buffer->state = EMPTY;
} else {
buffer->state = FULL;
}
pthread_cond_signal(&buffer->empty); // 醒通知消费者
pthread_mutex_unlock(&buffer->lock);
}
return NULL;
}
void* consumer(void* arg) {
Buffer* buffer = (Buffer*)arg;
int i;
while (1) {
pthread_mutex_lock(&buffer->lock);
while (buffer->count == 0) { // 如果缓冲区为空,等待
pthread_cond_wait(&buffer->empty, &buffer->lock);
}
// 消费数据
printf("Consumed: %d\n", buffer->data[buffer->count - 1]);
buffer->count--;
if (buffer->count == BUFFER_SIZE - 1) { // 设置状态变为满
buffer->state = FULL;
} else {
buffer->state = EMPTY;
}
pthread_cond_signal(&buffer->full); // 醒通知生产者
pthread_mutex_unlock(&buffer->lock);
}
return NULL;
}
int main() {
Buffer buffer;
for (int i = 0; i < BUFFER_SIZE; ++i) {
buffer.data[i] = i;
}
buffer.count = BUFFER_SIZE;
buffer.state = EMPTY;
pthread_mutex_init(&buffer.lock, NULL);
pthread_cond_init(&buffer.empty, NULL);
pthread_cond_init(&buffer.full, NULL);
pthread_t producer_thread, consumer_thread;
pthread_create(&producer_thread, NULL, producer, &buffer);
pthread_create(&consumer_thread, NULL, consumer, &buffer);
pthread_join(producer_thread, NULL);
pthread_join(consumer_thread, NULL);
pthread_mutex_destroy(&buffer.lock);
pthread_cond_destroy(&buffer.empty);
pthread_cond_destroy(&buffer.full);
return 0;
}
```
阅读全文
相关推荐
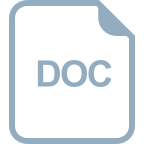
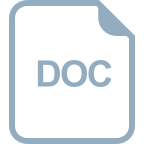
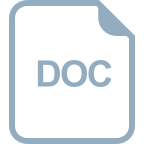

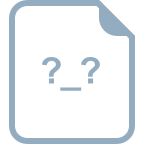
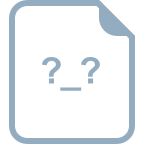
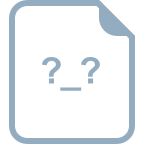
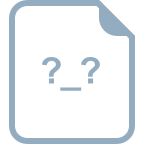
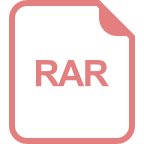
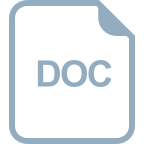
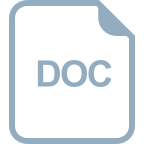
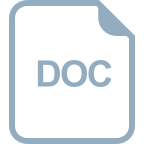





