用python完成树莓派温湿度的检测
时间: 2023-06-08 11:03:45 浏览: 129
很高兴回答你的问题!对于树莓派温湿度检测,我们可以使用DHT11或DHT22传感器来实现。使用Python编写程序,通过GPIO口读取传感器数据,并进行相应的处理和显示。以下是示例程序:
```python
import Adafruit_DHT
sensor = Adafruit_DHT.DHT11 # 设置传感器类型
pin = 4 # 设置GPIO口
humidity, temperature = Adafruit_DHT.read_retry(sensor, pin) # 读取传感器数据
if humidity is not None and temperature is not None:
print('温度:{:.1f} 度,湿度:{:.1f}%'.format(temperature, humidity))
else:
print('读取数据失败')
```
注意,要安装 Adafruit_DHT 库,可以使用以下命令进行安装:
```shell
sudo apt-get update
sudo apt-get install python3-pip
sudo python3 -m pip install Adafruit_DHT
```
相关问题
树莓派dht11温湿度检测
要在树莓派上使用DHT11传感器,首先需要安装相应的Python库。可以使用以下命令安装:
```
sudo apt-get update
sudo apt-get install python3-pip
sudo python3 -m pip install Adafruit_DHT
```
安装完成后,可以使用以下代码在Python中读取DHT11传感器的温度和湿度数据:
```python
import Adafruit_DHT
# 设置传感器类型和引脚号
sensor = Adafruit_DHT.DHT11
pin = 4
# 读取传感器数据
humidity, temperature = Adafruit_DHT.read_retry(sensor, pin)
# 输出数据
if humidity is not None and temperature is not None:
print('Temperature={0:0.1f}*C Humidity={1:0.1f}%'.format(temperature, humidity))
else:
print('Failed to read sensor data')
```
在以上代码中,传感器类型为DHT11,引脚号为4。`read_retry`方法会尝试多次读取传感器数据,直到成功或达到最大尝试次数。如果读取成功,将会输出温度和湿度数据。如果读取失败,则会输出错误信息。
注意,在使用DHT11传感器时,需要将传感器的VCC引脚连接到树莓派的5V引脚,GND引脚连接到树莓派的GND引脚,DATA引脚连接到树莓派的GPIO引脚。另外,为了减少读取传感器数据时的干扰,建议在DATA引脚和树莓派GPIO引脚之间加上一个10K欧姆的上拉电阻。
写出树莓派温湿度和烟雾蜂鸣器报警代码
以下是一个基本的树莓派温湿度和烟雾蜂鸣器报警代码:
```python
import RPi.GPIO as GPIO
import Adafruit_DHT
# 设置GPIO模式为BCM
GPIO.setmode(GPIO.BCM)
# 设置传感器类型和引脚
humidity_sensor = Adafruit_DHT.DHT11
humidity_pin = 4
# 设置烟雾传感器引脚
smoke_pin = 17
# 设置蜂鸣器引脚
buzzer_pin = 27
# 初始化蜂鸣器引脚
GPIO.setup(buzzer_pin, GPIO.OUT)
# 持续监测温湿度和烟雾
while True:
# 读取温湿度
humidity, temperature = Adafruit_DHT.read_retry(humidity_sensor, humidity_pin)
if humidity is not None and temperature is not None:
print('温度: {:.1f}℃, 湿度: {:.1f}%'.format(temperature, humidity))
else:
print('无法获取温湿度数据!')
# 读取烟雾传感器数据
smoke = GPIO.input(smoke_pin)
if smoke == GPIO.HIGH:
print('检测到烟雾!')
# 触发蜂鸣器
GPIO.output(buzzer_pin, GPIO.HIGH)
else:
# 关闭蜂鸣器
GPIO.output(buzzer_pin, GPIO.LOW)
```
需要注意的是,这只是一个基本的示例代码。如果要使用在实际场景中,需要根据具体情况进行修改和优化。例如,可以添加发送邮件或短信通知等功能,以及设置温度和湿度的警戒值等。
相关推荐
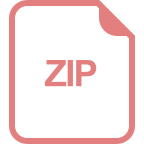
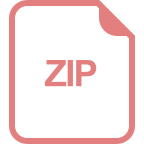
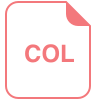
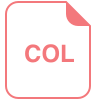










