利用遗传算法编写有多个货车的VRP问题的代码
时间: 2023-08-13 18:03:44 浏览: 98
首先,需要明确一下VRP问题的定义:VRP(Vehicle Routing Problem)是指在一个有限的车队和一些需要配送的点之间,如何规划车辆的路径,使得所有需要配送的点都被服务到,且在满足车辆的容量和路程限制的前提下,使得总配送成本最小。
下面是一个基于遗传算法的多车VRP问题的代码实现(以Python为例):
```python
import random
# 定义问题参数
num_customers = 10 # 客户数量
num_vehicles = 2 # 车辆数量
vehicle_capacity = 20 # 车辆容量
depot = 0 # 起点
# 定义遗传算法参数
population_size = 50 # 种群大小
mutation_rate = 0.1 # 变异率
elite_rate = 0.2 # 精英率
generations = 100 # 迭代次数
# 生成随机的初始解
def generate_random_solution():
solution = []
for i in range(num_vehicles):
route = [depot]
capacity_remaining = vehicle_capacity
for j in range(num_customers):
if capacity_remaining >= demands[j]:
route.append(j)
capacity_remaining -= demands[j]
route.append(depot)
solution.append(route)
return solution
# 计算路径长度
def calculate_distance(route):
distance = 0
for i in range(len(route)-1):
distance += distances[route[i]][route[i+1]]
return distance
# 计算解的总路径长度
def calculate_solution_distance(solution):
distance = 0
for route in solution:
distance += calculate_distance(route)
return distance
# 交叉操作
def crossover(parent1, parent2):
child1 = []
child2 = []
cut_point = random.randint(1, num_customers-1)
for i in range(num_vehicles):
if i % 2 == 0:
child1.append(parent1[i][:cut_point] + parent2[i][cut_point:])
child2.append(parent2[i][:cut_point] + parent1[i][cut_point:])
else:
child1.append(parent2[i][:cut_point] + parent1[i][cut_point:])
child2.append(parent1[i][:cut_point] + parent2[i][cut_point:])
return child1, child2
# 变异操作
def mutate(solution):
for i in range(num_vehicles):
if random.random() < mutation_rate:
route = solution[i]
for j in range(1, len(route)-1):
if random.random() < mutation_rate:
k = random.randint(1, len(route)-2)
route[j], route[k] = route[k], route[j]
solution[i] = route
return solution
# 选择操作
def select(population):
sorted_population = sorted(population, key=lambda x: calculate_solution_distance(x))
elite_size = int(elite_rate * population_size)
elite = sorted_population[:elite_size]
selection_probs = [i/sum(range(1, population_size+1)) for i in range(1, population_size+1)]
selected = random.choices(population, weights=selection_probs, k=population_size-elite_size)
selected.extend(elite)
return selected
# 初始化距离矩阵和需求量数组
distances = []
demands = []
for i in range(num_customers+1):
row = []
for j in range(num_customers+1):
row.append(random.randint(1, 10))
distances.append(row)
if i != 0:
demands.append(random.randint(1, 5))
# 运行遗传算法
population = [generate_random_solution() for i in range(population_size)]
for generation in range(generations):
population = select(population)
next_generation = []
for i in range(int(population_size/2)):
parent1, parent2 = random.choices(population, k=2)
child1, child2 = crossover(parent1, parent2)
next_generation.extend([mutate(child1), mutate(child2)])
population = next_generation
best_solution = min(population, key=lambda x: calculate_solution_distance(x))
print("Best solution:", best_solution)
print("Total distance:", calculate_solution_distance(best_solution))
```
该代码通过遗传算法来寻找多车VRP问题的最优解。其中,`generate_random_solution`函数用于生成随机的初始解;`calculate_distance`函数用于计算单条路径的长度;`calculate_solution_distance`函数用于计算整个解的总路径长度;`crossover`函数用于进行交叉操作;`mutate`函数用于进行变异操作;`select`函数用于进行选择操作。在主函数中,首先初始化距离矩阵和需求量数组,然后运行遗传算法找到最优解并输出结果。
阅读全文
相关推荐
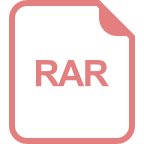
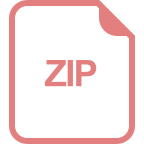
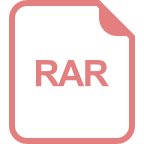
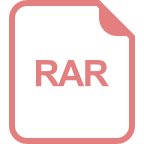
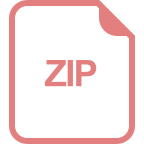
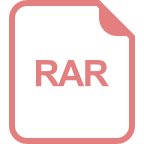
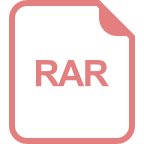
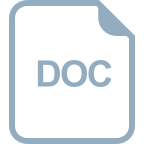
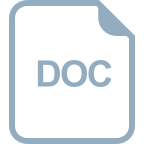
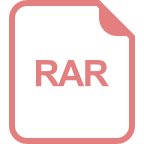
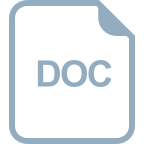
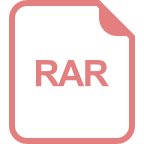
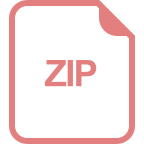
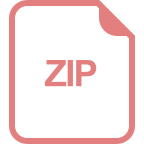
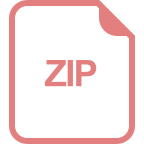