用C语言编写一个程序,输入 5个学生的学号、姓名、3 门课程的成绩和总分,求出总分最高的学生姓名并输出。并用二维表打印出来
时间: 2024-02-21 22:00:00 浏览: 35
好的,这是一个经典的 C 语言编程题,我来给您讲一下思路。
首先,我们可以定义一个结构体来存储每个学生的信息,包括学号、姓名、3 门课程的成绩和总分。结构体定义如下:
```c
struct student {
int id;
char name[20];
int score1, score2, score3;
int total;
};
```
接下来,我们可以定义一个数组来存储 5 个学生的信息,以及一个变量来记录总分最高的学生的下标。代码如下:
```c
int main() {
struct student stu[5];
int max_index = 0;
// 输入每个学生的信息并计算总分
for (int i = 0; i < 5; i++) {
// 输入学号、姓名和成绩
printf("请输入第 %d 个学生的学号、姓名和成绩:\n", i+1);
scanf("%d %s %d %d %d", &stu[i].id, stu[i].name, &stu[i].score1, &stu[i].score2, &stu[i].score3);
// 计算总分
stu[i].total = stu[i].score1 + stu[i].score2 + stu[i].score3;
// 更新总分最高的学生的下标
if (stu[i].total > stu[max_index].total) {
max_index = i;
}
}
// 输出总分最高的学生的姓名和成绩
printf("总分最高的学生是 %s,总分为 %d 分。\n", stu[max_index].name, stu[max_index].total);
// 用二维表打印学生信息
printf("学号\t姓名\t成绩1\t成绩2\t成绩3\t总分\n");
for (int i = 0; i < 5; i++) {
printf("%d\t%s\t%d\t%d\t%d\t%d\n", stu[i].id, stu[i].name, stu[i].score1, stu[i].score2, stu[i].score3, stu[i].total);
}
return 0;
}
```
运行程序,输入每个学生的信息,程序会自动计算总分,并输出总分最高的学生的姓名和成绩,并用二维表形式打印出所有学生的信息。
相关推荐
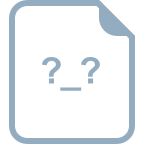














